java后端httpclient post提交文件流 及服务端接收文件流
时间: 2023-07-19 20:01:54 浏览: 63
### 回答1:
在Java后端,使用HttpClient库进行POST请求提交文件流,包括以下步骤:
1. 导入HttpClient库的依赖:
```xml
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.5.13</version>
</dependency>
```
2. 创建HttpClient对象:
```java
CloseableHttpClient httpClient = HttpClients.createDefault();
```
3. 创建HttpPost对象,并设置URL和请求体:
```java
HttpPost httpPost = new HttpPost("http://example.com/upload");
File file = new File("path/to/file");
MultipartEntityBuilder builder = MultipartEntityBuilder.create();
builder.addPart("file", new FileBody(file));
HttpEntity multipart = builder.build();
httpPost.setEntity(multipart);
```
4. 执行POST请求并获取响应:
```java
CloseableHttpResponse response = httpClient.execute(httpPost);
```
在服务端接收文件流的代码如下:
1. 创建Spring Boot Controller并监听指定URL:
```java
@RestController
public class FileUploadController {
@PostMapping("/upload")
public String handleFileUpload(@RequestParam("file") MultipartFile file) {
// 处理文件流
// ...
return "File uploaded successfully.";
}
}
```
2. 接收到请求时,Spring框架会自动将文件流绑定到`MultipartFile`对象,并调用`handleFileUpload`方法进行处理。
以上就是使用HttpClient库进行Java后端HTTP POST请求提交文件流以及服务端接收文件流的简单示例。
### 回答2:
在Java后端中使用HttpClient进行post请求提交文件流的步骤如下:
1. 引入相关的依赖,如Apache HttpClient:
```xml
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.5.13</version>
</dependency>
```
2. 创建HttpClient对象:
```java
CloseableHttpClient httpClient = HttpClients.createDefault();
```
3. 创建HttpPost请求对象:
```java
HttpPost httpPost = new HttpPost(url);
```
其中,`url`为服务端接收文件流的接口地址。
4. 创建File实例或通过其他方式获取要上传的文件流:
```java
File file = new File("path/to/file");
FileBody fileBody = new FileBody(file);
```
5. 创建HttpEntity,并将文件对象添加到其中:
```java
MultipartEntityBuilder builder = MultipartEntityBuilder.create();
builder.addPart("file", fileBody);
HttpEntity httpEntity = builder.build();
```
6. 设置请求的Entity为刚创建的HttpEntity:
```java
httpPost.setEntity(httpEntity);
```
7. 发送post请求并获取响应:
```java
CloseableHttpResponse httpResponse = httpClient.execute(httpPost);
HttpEntity responseEntity = httpResponse.getEntity();
```
以上是使用HttpClient在Java后端进行post请求提交文件流的基本步骤。
在服务端接收文件流的代码示例如下(以Spring Boot为例):
1. 创建用于接收文件流的接口:
```java
@PostMapping("/upload")
public void uploadFile(MultipartFile file) {
// 处理文件流的业务逻辑
}
```
2. 在接口中使用MultipartFile对象接收文件流,Spring Boot会自动将上传的文件流封装成MultipartFile对象。
3. 可以在接口中进行一些与文件相关的处理,如保存文件、读取文件内容等。
以上就是使用Java后端的HttpClinet进行文件流提交以及在服务端接收文件流的简单介绍。
相关推荐
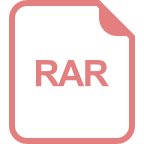














