用verilog HDL设计一个8位加法器,该加法器需考虑低位进位(cin)和向高位进位(cout)功能。
时间: 2024-12-23 15:25:01 浏览: 7
在Verilog HDL中设计一个8位加法器,你可以采用全加法器(Full Adder)的基本构建单元,并利用级联的方式来构建。以下是一个基本的设计思路:
1. **定义模块**:首先创建一个名为`full_adder`的模块,接受两个8位输入(A、B),一个低位进位输入(cin),以及两个输出端口(S、Cout),分别代表加法结果和进位。
```verilog
module full_adder (
input wire [7:0] A,
input wire [7:0] B,
input wire cin,
output wire [7:0] S,
output reg cout
);
```
2. **全加器结构**:全加器由三个门电路组成,包括XOR、AND、OR运算。将它们连接起来形成一个加法过程。
```verilog
// XOR gate for sum
wire xor_sum;
// AND gate for carry_in and A
wire and_a_cin;
// OR gate for carry_in, B, and AND(a, cin)
wire or_cin_b_and;
assign xor_sum = A ^ B;
assign and_a_cin = A & cin;
assign or_cin_b_and = cin | B;
// Compute sum (S) and carry out (cout)
assign S = {xor_sum[6:0], and_a_cin};
assign cout = or_cin_b_and;
```
3. **多级加法器**:为了得到8位的总和,你需要将多个全加器串联起来,每个全加器的低位进位cin连接到下一个全加器的高位进位cin。同时,最后一个全加器的cout连接到最终结果。
```verilog
module adder_8bit (
input wire [7:0] A,
input wire [7:0] B,
input wire cin,
output wire [7:0] sum,
output reg cout
);
full_adder fa0(A[7], B[7], cin, sum[7], cout);
full_adder fa1(A[6], B[6], fa0.cout, sum[6], cout);
// Repeat for all 8 bits...
// Connect the carry outputs from each stage
wire [7:0] intermediate_couts;
intermediate_couts = {fa1.cout, fa2.cout, ..., fa7.cout};
// Last stage of carry out is the final Cout
assign cout = intermediate_couts[7];
```
阅读全文
相关推荐
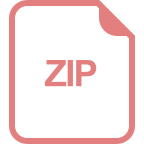
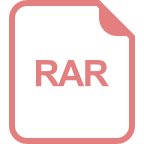
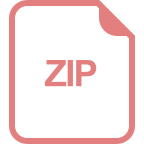
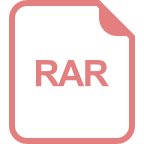
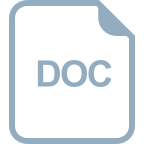
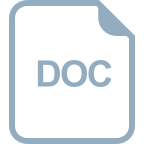
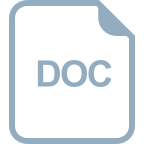
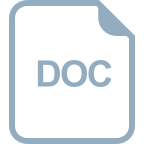
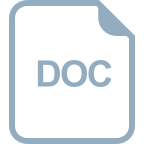
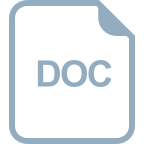
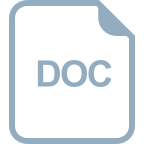
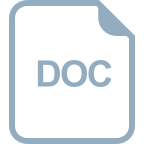
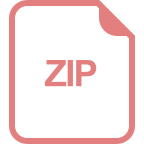