用c语言数组写从键盘输人字符串s和v,在字符串s中的最小元素后面插入字符串b。编写 一程序,将两个一维数组中对应元素的值相减后显示出来。
时间: 2024-10-01 12:08:57 浏览: 33
在C语言中,你可以使用`scanf`函数从键盘输入字符串`s`, `v`以及字符数组`b`,然后遍历这两个数组,找到并插入字符串`b`到`s`中的最小元素之后。接着,你可以创建一个新的数组来存储结果,对于每个对应位置的元素,做减法操作并将结果存入新数组。最后,打印出这个新的数组。
下面是一个简单的示例程序:
```c
#include <stdio.h>
#include <string.h>
#define MAX_LEN 100
void insertAfterMin(char s[], char b[]) {
int min_index = -1;
int i = 0;
// 找到s中最小元素的索引
while (i < strlen(s) && min_index == -1) {
if (min_index == -1 || s[i] < s[min_index]) {
min_index = i;
}
i++;
}
// 插入b到s中的最小元素后面
if (min_index != -1) {
memmove(&s[min_index + strlen(b)], &s[min_index], strlen(s) - min_index);
strcpy(&s[min_index], b);
} else {
printf("No minimum element found in the string.\n");
}
}
// 函数用于计算并显示数组元素之差
void displayDifference(int a[], char s[], int n) {
for (int i = 0; i < n; i++) {
int diff = a[i] - s[i];
printf("Element difference at index %d is: %d\n", i, diff);
}
}
int main() {
char s[MAX_LEN], v[MAX_LEN], b[5]; // 假设b的最大长度不超过4个字符
int a[MAX_LEN];
printf("Enter string s: ");
fgets(s, MAX_LEN, stdin); // 防止换行符
s[strlen(s) - 1] = '\0'; // 移除末尾的换行符
printf("Enter string v: ");
fgets(v, MAX_LEN, stdin);
v[strlen(v) - 1] = '\0';
printf("Enter character array b: ");
fgets(b, sizeof(b), stdin);
b[strlen(b) - 1] = '\0';
// 输入整数数组a
for (int i = 0; i < MAX_LEN; i++) {
scanf("%d", &a[i]);
}
// 计算并显示差异
insertAfterMin(s, b);
displayDifference(a, s, MAX_LEN);
return 0;
}
```
阅读全文
相关推荐
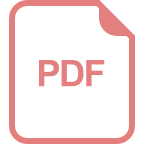
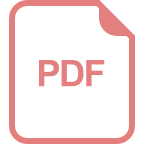
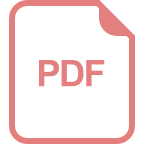





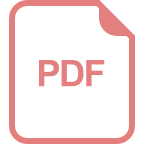
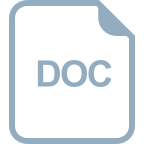







