定义点类和矩形类,将矩形类定义成组合类,生成矩形类的对象并对对象进行操作。计算矩形的周长和面积。(边长:6 和7;15和25)java代码实现
时间: 2024-11-25 12:16:43 浏览: 15
在Java中,我们可以首先定义一个Point类表示二维坐标,然后创建Rectangle类作为组合类,其中包含一个Point对象用于保存矩形的位置。下面是简单的代码实现:
```java
// 点类 Point
class Point {
double x;
double y;
public Point(double x, double y) {
this.x = x;
this.y = y;
}
// 获取和设置坐标的方法
public double getX() { return x; }
public double getY() { return y; }
public void setX(double x) { this.x = x; }
public void setY(double y) { this.y = y; }
}
// 矩形类 Rectangle (组合类)
class Rectangle extends Point {
private double width;
private double height;
public Rectangle(Point position, double width, double height) {
super(position.getX(), position.getY());
this.width = width;
this.height = height;
}
// 计算周长和面积的方法
public double perimeter() {
return 2 * (getWidth() + getHeight());
}
public double area() {
return getWidth() * getHeight();
}
public double getWidth() { return width; }
public double getHeight() { return height; }
public void setSize(double width, double height) {
this.width = width;
this.height = height;
}
}
// 主函数
public class Main {
public static void main(String[] args) {
// 创建两个矩形对象,分别表示边长为(6, 7)和(15, 25)
Rectangle rectangle1 = new Rectangle(new Point(0, 0), 6, 7);
Rectangle rectangle2 = new Rectangle(new Point(10, 10), 15, 25);
// 对对象进行操作并打印结果
System.out.println("Rectangle 1 properties:");
System.out.printf("Position: (%f, %f)\n", rectangle1.getX(), rectangle1.getY());
System.out.println("Perimeter: " + rectangle1.perimeter());
System.out.println("Area: " + rectangle1.area());
System.out.println("\nRectangle 2 properties:");
System.out.printf("Position: (%f, %f)\n", rectangle2.getX(), rectangle2.getY());
System.out.println("Perimeter: " + rectangle2.perimeter());
System.out.println("Area: " + rectangle2.area());
}
}
```
这个例子中,我们创建了两个矩形对象,并通过它们的宽和高计算了各自的周长和面积。注意,每个矩形都有自己的位置信息,这是通过继承`Point`类并结合组合得到的。
阅读全文
相关推荐
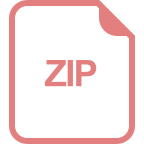
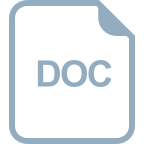

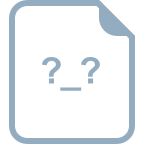
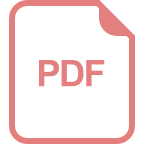
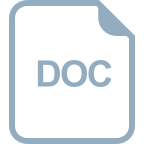
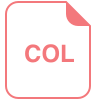
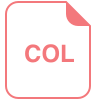
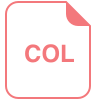
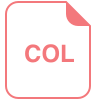

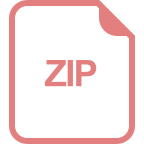
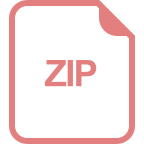
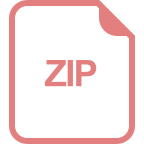