C语言 实现PBKDF2(Password-Based Key Derivation Function 2)哈希算法,其中SHA-256是哈希函数,迭代次数(iterations)1000
时间: 2024-10-12 14:04:38 浏览: 40
PBKDF2(Password-Based Key Derivation Function 2)是一种广泛用于密码安全存储的加密散列函数,通过增加迭代次数来提高安全性。以下是使用C语言实现PBKDF2(使用SHA-256哈希函数和1000次迭代)的一个简单示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/bio.h>
#include <openssl/pem.h>
#include <openssl/evp.h>
#include <openssl/hmac.h>
#define PBKDF2_ITERATIONS 1000
#define SHA256_DIGEST_LENGTH 32
void pbkdf2(const char *password, const char *salt, unsigned char *derived_key, size_t key_len) {
const EVP_MD *md = EVP_sha256();
unsigned char hash[SHA256_DIGEST_LENGTH];
int i;
HMAC(EVP_md_CTX_create(), password, strlen(password), salt, strlen(salt), hash, NULL);
for (i = 1; i <= PBKDF2_ITERATIONS; ++i) {
HMAC_update(EVP_md_CTX_create(), hash, sizeof(hash));
}
HMAC_final(EVP_md_CTX_create(), derived_key, &key_len);
}
int main() {
char password[] = "your_password";
char salt[] = "your_salt"; // Ideally, use a random salt
unsigned char derived_key[SHA256_DIGEST_LENGTH];
if (pbkdf2(password, salt, derived_key, sizeof(derived_key)) != 1) {
printf("Error: PBKDF2 failed.\n");
return -1;
}
printf("Derived Key: ");
for (size_t i = 0; i < SHA256_DIGEST_LENGTH; ++i) {
printf("%02x", derived_key[i]);
}
printf("\n");
return 0;
}
```
这个例子中,我们首先创建了一个`HMAC`上下文,并初始化了SHA-256哈希函数。然后对给定的密码和盐进行多次哈希操作(这里是1000次),最后将结果保存到`derived_key`数组中。
阅读全文
相关推荐
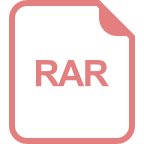
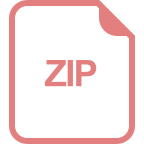
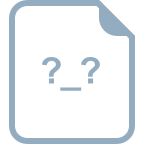

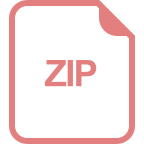
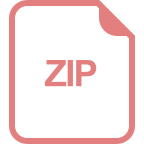
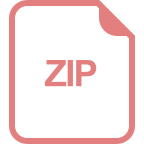
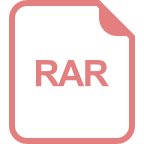
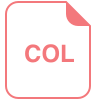
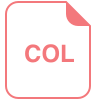
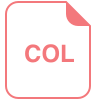
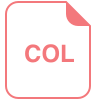
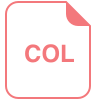
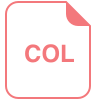
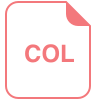



