vue 内容超出一屏显示 底部固定按钮
时间: 2023-08-31 12:03:05 浏览: 118
在Vue中实现内容超出一屏显示底部固定按钮,我们可以借助CSS样式和Vue的特性来实现。
首先,我们可以使用CSS的flex布局来创建一个容器,设置其flex-direction为列布局,并将高度设置为100vh,这样容器将占满整个屏幕。然后,我们在容器内部创建内容区域和底部固定按钮区域。
在内容区域中,我们可以使用Vue的v-for指令来遍历数据列表,并将每个数据项渲染为相应的内容组件。当内容超出一屏后,我们可以设置内容区域的overflow-y为auto,这样内容将会出现滚动条。
在底部固定按钮区域,我们可以使用CSS的position属性将按钮固定在底部,例如position: fixed; bottom: 0;。另外,为了避免底部按钮遮挡内容区域,可以设置内容区域的padding-bottom值等于底部按钮占据的高度。
最后,我们可以利用Vue的响应式特性来动态控制底部固定按钮的显示与隐藏。例如,可以通过监测内容区域的滚动事件,在内容滚动到底部时显示按钮,否则隐藏按钮。
综上所述,我们可以通过CSS样式和Vue特性来实现内容超出一屏显示底部固定按钮。这样,当页面内容超过一屏时,用户可以通过滚动来查看全部内容,并可以随时点击底部按钮进行其他操作。
相关问题
用vue写一个大屏显示系统
好的。为了使用 Vue 写一个大屏显示系统,您需要执行以下步骤:
1. 安装 Vue 和相关工具。首先,您需要安装 Node.js 和 npm,然后使用 npm 安装 Vue 及其相关工具,如 Vue CLI 和 Vue Router。
2. 创建一个新项目。使用 Vue CLI 创建一个新的 Vue 项目。此命令将创建一个带有默认模板的 Vue 项目。
3. 编写代码。接下来,您可以编写代码来实现您的大屏显示系统。可以使用 Vue 组件来组织代码,并使用 Vuex 来管理状态。
4. 在浏览器中查看结果。最后,使用 Vue CLI 启动开发服务器,然后在浏览器中打开网址即可查看结果。
希望这些信息对您有所帮助。如果您有任何其他问题,请随时发问。
vue实现按钮在屏幕的固定位置可以拖动
要实现按钮在屏幕的固定位置并且可拖动,需要使用Vue的指令和事件绑定。具体实现步骤如下:
1. 在Vue组件中定义一个按钮元素,并设置其样式为固定定位,例如:
```
<template>
<button class="fixed-btn" v-draggable>按钮</button>
</template>
<style>
.fixed-btn {
position: fixed;
bottom: 20px;
right: 20px;
}
</style>
```
2. 定义一个自定义指令v-draggable用来实现按钮的拖拽功能,例如:
```
Vue.directive('draggable', {
bind: function (el, binding, vnode) {
el.style.position = 'absolute';
el.style.cursor = 'move';
el.addEventListener('mousedown', function (e) {
let startX = e.clientX - el.offsetLeft;
let startY = e.clientY - el.offsetTop;
document.addEventListener('mousemove', move);
document.addEventListener('mouseup', stop);
function move (e) {
el.style.left = e.clientX - startX + 'px';
el.style.top = e.clientY - startY + 'px';
}
function stop () {
document.removeEventListener('mousemove', move);
document.removeEventListener('mouseup', stop);
}
});
}
});
```
3. 在组件中引入该指令,并在按钮元素上使用该指令即可实现按钮的拖拽功能。
```
<template>
<button class="fixed-btn" v-draggable>按钮</button>
</template>
```
这样按钮就可以在屏幕上固定位置并且可拖动了。需要注意的是,该功能只是实现了按钮的拖拽,如果需要将按钮的位置保存到后端或者在刷新页面后仍然保持按钮的位置,需要在拖拽结束时将按钮的位置信息发送到后端或者使用本地存储或Cookie等技术来保存位置信息。
相关推荐













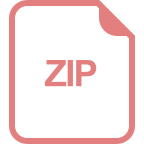
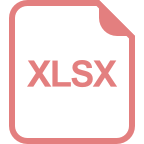
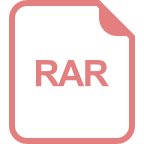