C++ map'及其用法
时间: 2024-09-09 08:03:56 浏览: 39
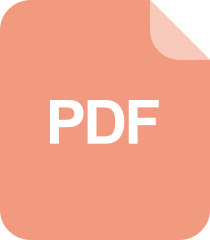
深入了解C++中map用法
`C++`中的`map`是关联容器,它存储的是键值对,按照键(key)的比较顺序排列。以下是一些基本的`map`用法:
1. **创建并初始化**[^2]:
```cpp
#include <map>
using namespace std;
int main() {
// 创建一个空map
map<int, string> m;
// 使用区间构造从已知键值对列表中构建map
vector<pair<int, string>> vec{{1, "one"}, {2, "two"}};
m(vec.begin(), vec.end());
return 0;
}
```
2. **查找元素**:
```cpp
// 查找键对应的值
if (m.find(1) != m.end()) {
cout << "Value for key 1: " << m[1] << endl;
}
// 如果键不存在,find返回map的end()
if (m.find(3) == m.end()) {
cout << "Key 3 not found." << endl;
}
```
3. **插入和更新**:
```cpp
// 插入新的键值对
m[3] = "three";
// 更新已有键的值
m[1] = "uno";
```
4. **删除元素**:
```cpp
// 删除指定键的元素
m.erase(1);
// 删除整个范围内的元素
for (auto it = m.begin(); it != m.end(); ++it) {
if (it->first > 2) {
m.erase(it);
break;
}
}
```
5. **遍历**:
```cpp
// 遍历map
for (const auto& pair : m) {
cout << "Key: " << pair.first << ", Value: " << pair.second << endl;
}
```
`unordered_map`, 又名哈希映射,虽然不保证元素的顺序,但查找速度通常更快,因为它是通过哈希表实现的,常用于需要快速查找的情况。
阅读全文
相关推荐
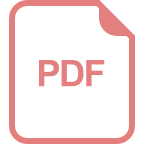
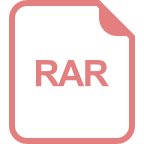















