arduino蓝牙控制风扇转速代码
时间: 2024-09-11 12:13:57 浏览: 88
在Arduino项目中,使用蓝牙模块如 HC-05 或 Nordic nRF52840 控制风扇转速,首先需要准备硬件包括Arduino Uno(或其他兼容板),蓝牙模块,以及一个直流电机驱动电路(例如步进电机或霍尔效应风扇)。以下是基本步骤和示例代码:
1. **硬件连接**:
- Arduino Uno接到蓝牙模块 TX 和 RX 端口
- 蓝牙模块接到电脑的串口(USB端口)
- 风扇连接到Arduino的数字输出引脚,通过限流电阻保护电机
2. **软件安装**:
- 安装arduino IDE 并添加蓝牙库支持,比如 "SoftwareSerial" 和 "FastLED"(如果用于控制RGB风扇)
3. **编写代码**:
```cpp
#include <SoftwareSerial.h>
#include <FastLED.h> // 如果使用RGB风扇,则需此库
// 假设你的蓝牙模块是 SoftwareSerial对象
SoftwareSerial ble(2, 3); // RX,TX针脚配置
const int fanPin = 9; // 风扇连接的GPIO
#define NUM_LEDS 3 // RGB风扇LED数量
CRGB leds[NUM_LEDS];
void setup() {
Serial.begin(9600);
ble.begin(9600);
FastLED::setup(NUM_LEDS, LED_TYPE_RGBW); // 初始化LED灯
}
void loop() {
if (ble.available()) { // 检查蓝牙接收数据
char command[10];
ble.read_until('\n', command); // 读取直到换行符
command[strlen(command) - 1] = '\0'; // 去除结尾的换行符
switch(command[0]) { // 根据命令控制风扇速度或颜色
case 'F':
setFanSpeed(command + 1);
break;
case 'C':
setFanColor();
break;
default:
Serial.println("Invalid command");
}
}
}
void setFanSpeed(int speed) {
int dutyCycle = map(speed, 0, 100, 0, 255); // 将输入的速度映射到0-255 PWM值
analogWrite(fanPin, dutyCycle);
}
void setFanColor() {
// 更新RGBW灯的颜色,这里仅做示例,实际应用需要处理接收到的颜色指令
for (int i = 0; i < NUM_LEDS; i++) {
leds[i] = CRGB::Of(colorValue, colorValue, colorValue); // 使用红、绿、蓝、白各色
}
updateLEDs(); // 发送颜色给LED
}
void updateLEDs() {
FastLED.show(); // 显示更新后的灯光
}
```
请注意,这个示例假设你在手机应用程序中发送字符 'F' 加上风扇速度(0-100)来控制风扇转速,或发送 'C' 来改变风扇的RGB颜色。
阅读全文
相关推荐
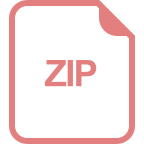
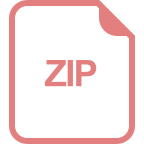
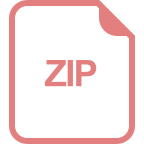
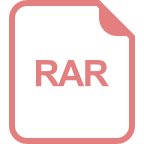
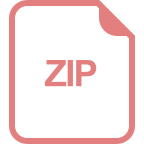
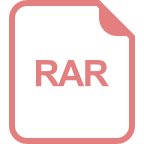
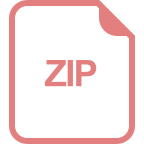
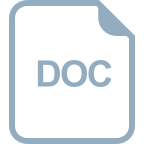
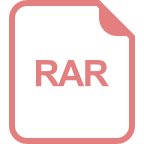
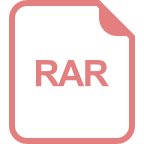
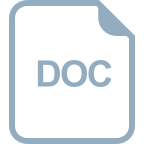
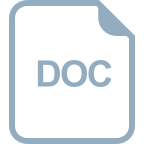
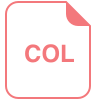
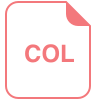
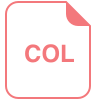
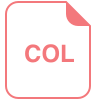

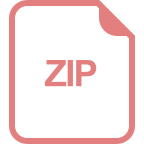