python保留有效数字
时间: 2024-09-28 20:05:16 浏览: 55
Python中的保留有效数字通常是指通过`round()`函数或科学计数法`"{:.n}`"来控制浮点数结果的小数位数。当你需要将一个数值四舍五入到特定小数位数时,可以这样做:
```python
# 使用 round() 函数
num = 3.141592653589793
rounded_num = round(num, 2) # 保留两位小数
print(rounded_num) # 输出: 3.14
# 或者使用 format() 方法
precision = 3
formatted_num = "{:.{prec}f}".format(num, prec=precision)
print(formatted_num) # 输出: 3.142 (如果 num 是正数,否则可能是 3.141)
# 使用 f-string (Python 3.6 及以上版本)
formatted_num = f"{num:.{precision}f}"
print(formatted_num)
```
注意,`round()`会直接截断多余的数字,而`"{:.n}"`会在最后填充零,直到指定的小数位数。
相关问题
python保留有效数字与保留小数
在Python中,保留有效数字和保留小数通常是指格式化浮点数以便在输出时控制其精度。有两种常用的方法:
1. 使用`round()`函数:这个内置函数可以对数值进行四舍五入到指定的小数位数。例如:
```python
num = 3.1415926
rounded_num = round(num, 2) # 保留两位小数
print(rounded_num) # 输出:3.14
```
2. `format()`函数:通过字符串格式化,可以更精确地控制小数位数和是否显示多余的零。示例:
```python
num = 0.0078125
formatted_num = "{:.2f}".format(num) # 保留两位小数,不足则补零
print(formatted_num) # 输出:0.01
```
3. `decimal`模块:如果需要更高精度的保留,可以使用`Decimal`类,它支持任意精度的小数计算:
```python
from decimal import Decimal
d = Decimal('0.0078125')
formatted_d = format(d, '.2f') # 同样保留两位小数
```
python 保留两位有效数字
可以使用 Python 中的内置函数 `round()` 来保留两位有效数字。例如,将一个浮点数 `x` 保留两位有效数字,可以使用以下代码:
```
x = 3.1415926
y = round(x, 2)
print(y)
```
输出结果为 `3.14`,即将原始浮点数保留了两位有效数字。
阅读全文
相关推荐
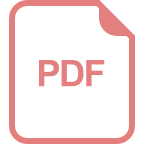
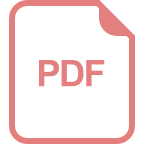
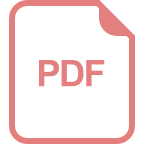













