1) 请你定义一个结构体用于存储日期(年、月、日)。 并编写函数(至少一个),函数可以接受两个日期结构体作为参数(传参方式不可以使用C++中的引用调用,其余方式不限制),计算它们之间的天数差距后,返回
时间: 2024-11-20 16:38:05 浏览: 10
在C++中,我们可以创建一个名为`Date`的结构体来存储日期信息,并包含三个成员变量:年份、月份和日子。然后我们定义一个名为`calculateDaysDifference`的函数,该函数接收两个`Date`类型的参数,通过计算它们之间相隔的天数来实现差值的计算。
```cpp
#include <iostream>
// 定义日期结构体
struct Date {
int year;
int month;
int day;
};
// 计算两个日期之间的天数差
int calculateDaysDifference(const Date& date1, const Date& date2) {
// 首先处理闰年的情况
bool isLeapYear(int year) {
return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
}
// 获取每个月的平均天数(假设所有月份都有30天)
int daysInMonth[13] = {31, 28, isLeapYear(date1.year) ? 29 : 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
// 计算天数差
int totalDays = 0;
for (int i = 0; i < date1.month; ++i) {
totalDays += daysInMonth[i];
}
totalDays += date1.day;
if (date2.month > date1.month) {
for (int i = date1.month + 1; i < date2.month; ++i) {
totalDays += daysInMonth[i];
}
} else if (date2.month == date1.month && date2.day > date1.day) {
totalDays++;
}
return abs(totalDays - (daysInMonth[date2.month - 1] + date2.day));
}
int main() {
Date date1 = {2023, 2, 28};
Date date2 = {2023, 3, 5};
int daysDiff = calculateDaysDifference(date1, date2);
std::cout << "The difference between the two dates is: " << daysDiff << " days.\n";
return 0;
}
```
在这个示例中,`calculateDaysDifference`函数首先确定了第一个日期之后的所有非闰年的月份总共有多少天,再加上第一个日期的实际天数。然后,如果第二个日期晚于第一个,它会加上第二个日期所在月份的剩余天数。最后,返回两个日期之间相差的天数。注意,这里没有处理二月特殊情况,因为题目没有明确指出需要考虑闰年的情况。在实际应用中,可以根据需求进行调整。
阅读全文
相关推荐
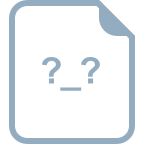
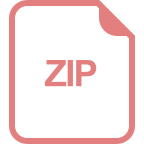
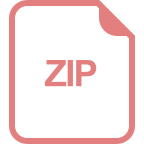

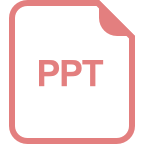
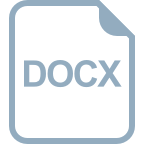
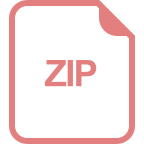
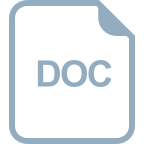
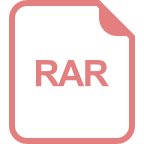
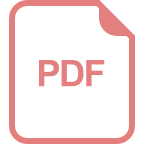
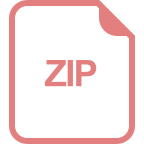
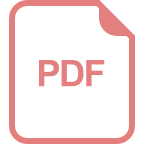
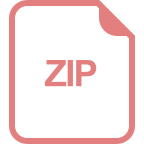
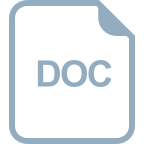
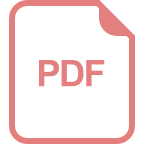
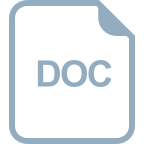
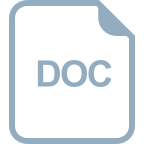