如何使用Pillow库生成彩色的爱心图片?
时间: 2024-09-21 14:07:57 浏览: 30
使用Pillow库生成彩色爱心图片的基本步骤如下:
1. 首先,你需要安装Pillow库,如果没有安装,可以使用pip install pillow命令进行安装。
2. 导入必要的模块:
```python
from PIL import Image, ImageDraw, ImageFont
```
3. 创建一个新的白色背景图片:
```python
img = Image.new('RGB', (size_x, size_y), 'white') # size_x和size_y是你要的图片宽度和高度
```
4. 创建一个ImageDraw对象,并设置填充色和笔刷:
```python
draw = ImageDraw.Draw(img)
fill_color = (color_r, color_g, color_b) # 这里是红(r), 绿(g), 蓝(b)的颜色值
brush_size = 10 # 爱心线条的粗细
```
5. 定义一个函数来画爱心,可以使用`draw.polygon()`或`draw.ellipse()`等方法,这里以椭圆为例:
```python
def draw_heart(centre, radius):
points = [(centre[0] - radius, centre[1]),
(centre[0] + radius, centre[1]),
(centre[0], centre[1] - radius),
(centre[0], centre[1] + radius)]
draw.ellipse(points, fill=fill_color)
# 设置爱心的位置和大小
centre = (img.width // 2, img.height // 2) # 中心位置
radius = min(img.width, img.height) / 3 # 椭圆半径,根据图片尺寸调整
# 绘制爱心
draw_heart(centre, radius)
```
6. 保存图片到文件:
```python
img.save("heart.png")
```
7. 如果你想给爱心添加文字,可以使用`ImageFont`模块配合`Text`对象:
```python
font = ImageFont.truetype('arial.ttf', font_size)
text = "Love"
text_position = (img.width // 4, img.height * 0.8)
img_draw_text = ImageDraw.Draw(img)
img_draw_text.text(text_position, text, fill='black')
```
完成以上步骤后,你就得到了一个彩色的爱心图片。
相关推荐
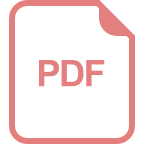
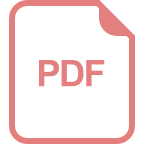














