C++编写一个能管理多条学生信息的程序。
时间: 2024-10-23 20:14:26 浏览: 10
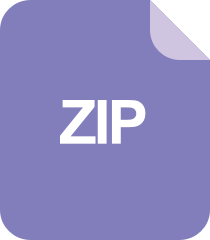
用C++编写的图书管理系统,采用txt文件存储图书信息和用户信息.zip
在C++中,你可以创建一个简单的程序来管理多条学生信息。首先,我们可以设计一个`Student`类,包含学生的姓名、学号和成绩等属性,然后创建一个容器(如`vector`)来存储这些学生对象。下面是一个简化的示例:
```cpp
#include <iostream>
#include <string>
#include <vector>
// 定义学生类
class Student {
public:
std::string name;
int id;
float score;
// 构造函数和获取setter/getter
Student(std::string n, int i, float s) : name(n), id(i), score(s) {}
std::string getName() const { return name; }
int getId() const { return id; }
float getScore() const { return score; }
// 用于打印学生信息
void display() {
std::cout << "Name: " << name << ", ID: " << id << ", Score: " << score << std::endl;
}
};
int main() {
// 创建一个学生信息列表
std::vector<Student> students;
// 添加新学生
students.push_back(Student("Alice", 1001, 85.5));
students.push_back(Student("Bob", 1002, 90.0));
// 管理学生信息
for (const auto& student : students) {
student.display();
}
// 查询和操作
std::string searchName = "Alice";
for (auto it = students.begin(); it != students.end(); ++it) {
if (it->getName() == searchName) {
std::cout << "Found student: " << it->getName() << "\n";
break;
}
}
return 0;
}
```
在这个程序中,我们创建了一个`Student`类,并使用`std::vector`存储了多个学生对象。通过遍历`students`容器,可以轻松地管理、显示或搜索学生信息。
阅读全文
相关推荐
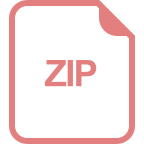
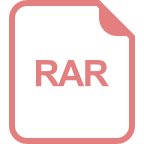


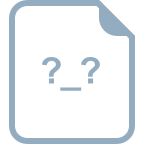
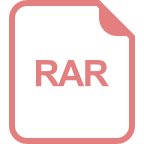
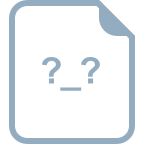
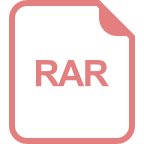
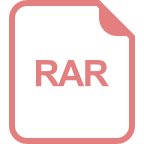
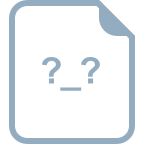
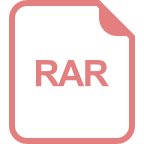
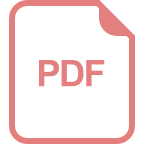
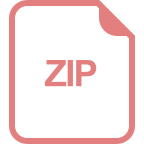
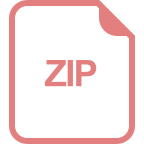
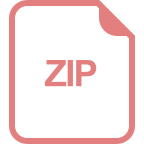
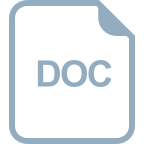
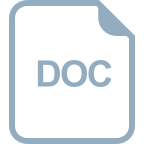
