如何用c语言输出字符串中特定位置的字符
时间: 2024-09-16 12:06:25 浏览: 64
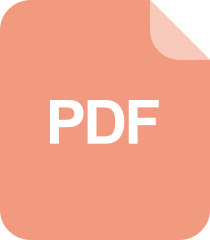
使用C语言解决字符串匹配问题的方法
在C语言中,要输出字符串中特定位置的字符,你可以使用数组索引来访问。字符串在C语言中是以字符数组的形式存储的,每个字符都有一个对应的索引,索引是从0开始的。下面是一个简单的例子,假设我们有一个名为`str`的字符数组:
```c
#include <stdio.h>
int main() {
char str[] = "Hello, World!";
int position; // 用户指定的位置
printf("请输入你要查询的字符位置:");
scanf("%d", &position); // 输入位置
// 确保索引不会超出字符串长度
if (position >= 0 && position < strlen(str)) {
char character = str[position]; // 获取指定位置的字符
printf("字符 '%c' 在字符串中的位置 %d:\n", character, position);
} else {
printf("无效的位置。\n");
}
return 0;
}
```
在这个示例中,我们首先获取用户输入的位置,然后检查这个位置是否有效(在0到字符串长度减一之间)。如果有效,就通过索引`position`获取对应位置的字符,并将其打印出来。
阅读全文
相关推荐
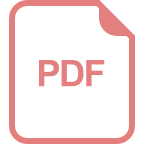
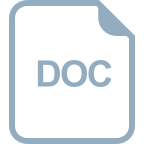


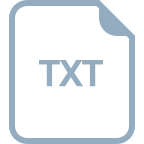
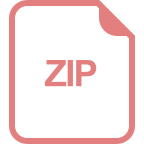
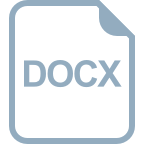
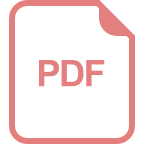
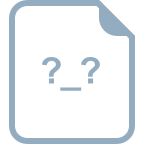
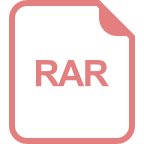
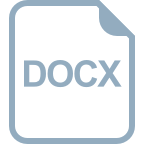
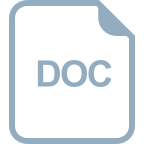
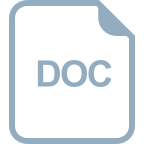
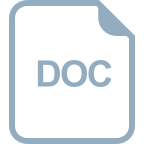
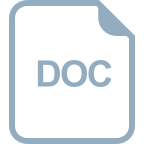
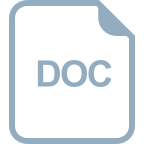
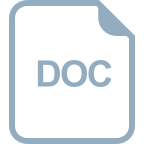