Linux系统中 怎么将float数组修改为自定义学生结构数组 完成5个学生信息初始化写入student文件,并读出前面两位数学生的信息打印至屏幕
时间: 2024-09-06 18:05:42 浏览: 42
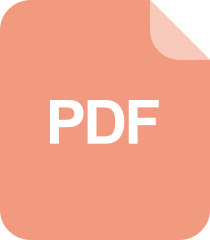
Python寻找两个有序数组的中位数实例详解
在Linux系统中,如果你有一个`float`类型的数组想要转换为自定义的`Student`结构体数组,首先你需要定义`Student`结构体并包含必要的成员(比如姓名、分数等)。假设`Student`结构体如下:
```c
#include <stdio.h>
#include <string.h>
typedef struct {
char name[50];
float score;
} Student;
void init_students(Student* students, int num_students) {
for (int i = 0; i < num_students; i++) {
// 初始化名字(这里假设名字固定)
strncpy(students[i].name, "Student", sizeof(students[i].name));
// 初始化分数,例如设置前两个学生的分数为0和1
if (i <= 2) {
students[i].score = i;
} else {
students[i].score = -1; // 其他学生设为无效值
}
}
}
void write_to_file(const char* filename, const Student* students, int num_students) {
FILE* file = fopen(filename, "w");
if (file != NULL) {
for (int i = 0; i < num_students; i++) {
fprintf(file, "%s %f\n", students[i].name, students[i].score);
}
fclose(file);
printf("Student information written to file successfully.\n");
} else {
perror("Error opening file.");
}
}
void read_and_print(const char* filename, Student* students, int num_students) {
FILE* file = fopen(filename, "r");
if (file != NULL) {
for (int i = 0; i < num_students && fscanf(file, "%[^ ]%f\n", students[i].name, &students[i].score) == 2; i++) {
printf("Name: %s Score: %.2f\n", students[i].name, students[i].score);
}
fclose(file);
} else {
perror("Error reading file.");
}
}
int main() {
Student students[5]; // 假设我们有5个学生的空间
init_students(students, 5);
write_to_file("student.txt", students, 5); // 写入学生信息到文件
read_and_print("student.txt", students, 5); // 打印文件中前两位学生的分数
return 0;
}
```
在这个示例中,我们首先定义了`Student`结构体,然后创建了一个`students`数组。`init_students`函数用于初始化学生信息,其中前两个学生分数赋值为0和1。接下来,我们将学生信息写入到`student.txt`文件,并从该文件中读取并打印出前两位学生的分数。
阅读全文
相关推荐
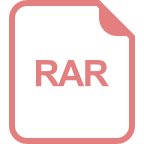
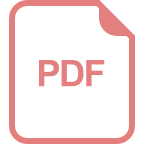
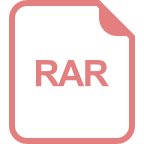
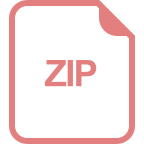
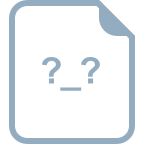
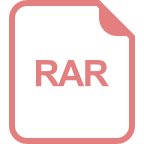
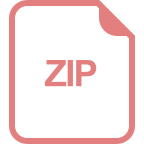
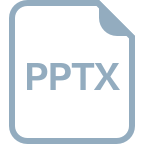
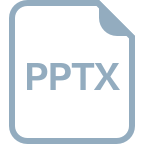
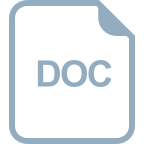
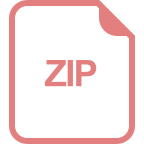
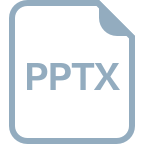
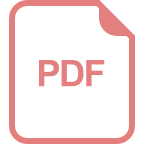
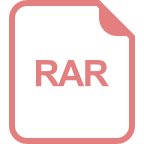
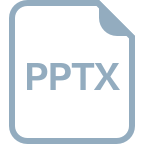
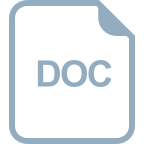
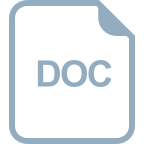