在Java中利用OpenCV库处理网络摄像头的RTSP流并将其保存为录像,能运行的代码
时间: 2024-10-08 21:15:51 浏览: 38
在Java中使用OpenCV库处理网络摄像头的RTSP流并保存为录像,首先需要安装OpenCV的Java bindings,然后可以按照以下步骤编写代码:
```java
import org.opencv.core.Core;
import org.opencv.core.Mat;
import org.opencv.highgui.HighGui;
import org.opencv.videoio.VideoCapture;
public class RTSPRecorder {
private static final String CAM_URL = "rtsp://your_camera_url"; // 替换为你的RTSP地址
private VideoCapture capture;
public static void main(String[] args) {
System.loadLibrary(Core.NATIVE_LIBRARY_NAME); // 加载OpenCV库
try {
RTSPRecorder recorder = new RTSPRecorder();
recorder.openCamera();
Mat frame = new Mat();
while (true) { // 循环读取帧并保存
if (!recorder.readFrame(frame)) break; // 如果读取失败,退出循环
HighGui.imwrite("output_" + System.currentTimeMillis() + ".jpg", frame); // 保存当前帧到本地文件
// 如果需要实时录像,可以用VideoWriter替换imwrite,如:
// VideoWriter writer = new VideoWriter("output.mp4", VideoWriter.fourcc('M', 'J', 'P', 'G'), 30, frame.size());
// writer.write(frame);
}
capture.release(); // 关闭摄像头
} catch (Exception e) {
e.printStackTrace();
}
}
private void openCamera() throws Exception {
capture = new VideoCapture(CAM_URL);
if (!capture.isOpened()) {
throw new Exception("Failed to open camera: " + CAM_URL);
}
}
private boolean readFrame(Mat frame) {
return capture.read(frame);
}
}
```
请注意,这个示例假设你已经在项目中包含了OpenCV库,并且RTSP服务器允许访问。`CAM_URL`应替换为你实际的RTSP流URL。
阅读全文
相关推荐
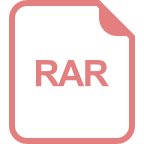
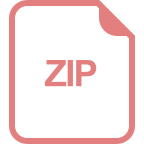
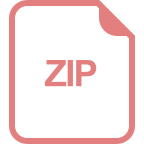



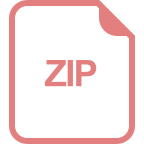
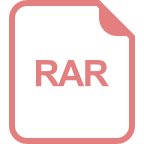
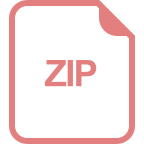
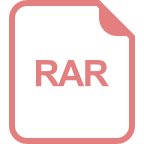
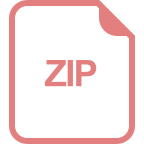
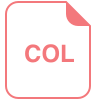
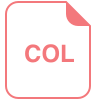
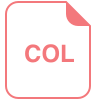
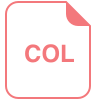
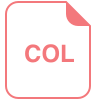
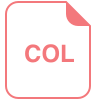

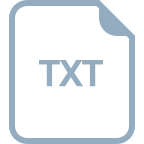