mainwindow.cpp:9:7: error: redefinition of 'MainWindow' mainwindow.h:10:7: note: previous definition is here
时间: 2024-04-15 07:29:33 浏览: 25
这个错误通常是由于在头文件和源文件中重复定义了类 `MainWindow` 导致的。
请确保 `mainwindow.h` 文件中只有类的声明,不包含类的定义。而在 `mainwindow.cpp` 文件中,包含类的定义和实现。
以下是一个修复该错误的示例:
mainwindow.h:
```cpp
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <QMainWindow>
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
MainWindow(QWidget *parent = nullptr);
~MainWindow();
};
#endif // MAINWINDOW_H
```
mainwindow.cpp:
```cpp
#include "mainwindow.h"
MainWindow::MainWindow(QWidget *parent)
: QMainWindow(parent)
{
// 构造函数的实现
}
MainWindow::~MainWindow()
{
// 析构函数的实现
}
```
请注意,在 `mainwindow.cpp` 中,我们只包含了 `mainwindow.h` 头文件,而不是重复定义类 `MainWindow`。
这样修改后,应该就可以解决 "redefinition of 'MainWindow'" 错误了。
相关问题
mainwindow.cpp:27:18: error: return type of out-of-line definition of 'MainWindow::selectFile' differs from that in the declaration mainwindow.h:22:9: note: previous declaration is here
根据你提供的错误信息,函数 `MainWindow::selectFile()` 的定义与其声明的返回类型不一致。
在你的 `mainwindow.h` 文件中,`MainWindow::selectFile()` 函数的声明的返回类型是 `int`,但是在 `mainwindow.cpp` 文件中的函数定义中,返回类型被修改为了 `bool`。
要修正这个问题,你需要确保函数的声明和定义的返回类型是一致的。根据你在 `selectFile()` 函数中的实现,返回类型应该是 `bool`。
将 `mainwindow.h` 文件中的函数声明修改为:
```cpp
bool selectFile();
```
修正后的代码如下所示:
**mainwindow.h**
```cpp
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <QMainWindow>
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
MainWindow(QWidget *parent = nullptr);
~MainWindow();
private slots:
bool selectFile();
private:
Ui::MainWindow *ui;
};
#endif // MAINWINDOW_H
```
**mainwindow.cpp**
```cpp
#include "mainwindow.h"
#include "ui_mainwindow.h"
#include <QDebug>
MainWindow::MainWindow(QWidget *parent)
: QMainWindow(parent)
, ui(new Ui::MainWindow)
{
QPushButton *selectButton = new QPushButton("选择文件", this);
connect(selectButton, &QPushButton::clicked, this, &MainWindow::selectFile);
QVBoxLayout *layout = new QVBoxLayout;
layout->addWidget(selectButton);
QWidget *centralWidget = new QWidget(this);
centralWidget->setLayout(layout);
setCentralWidget(centralWidget);
ui->setupUi(this);
}
MainWindow::~MainWindow()
{
delete ui;
}
bool MainWindow::selectFile()
{
QString dir = QFileDialog::getOpenFileName(this, "选择文件", "", "DWG Files (*.dwg)");
if (dir.isEmpty()) {
qDebug() << "error: File import failure!";
return false;
} else {
// 进行导入操作
// ...
return true;
}
}
```
请确保在 `mainwindow.cpp` 文件中包含了 `#include "mainwindow.h"` 的代码行。这样可以确保函数的定义与声明是一致的。
mainwindow.cpp:9:10: 'QTextCodec' file not found
如果在编译时出现 'QTextCodec' 文件找不到的错误,可能是因为 Qt 5.14 及更高版本中已经删除了 `QTextCodec` 类。Qt 5.14 引入了新的字符编码支持,使用 `QString` 和 `QByteArray` 直接处理字符编码,而不再需要 `QTextCodec`。
如果你使用的是 Qt 5.14 或更高版本,可以使用以下代码将 UTF-8 编码的字符串转换为 GBK 编码:
```cpp
QString utf8String = "UTF-8 编码的字符串";
QByteArray utf8Bytes = utf8String.toUtf8();
QTextCodec *codec = QTextCodec::codecForName("GBK");
QString gbkString = codec->toUnicode(utf8Bytes);
```
如果你使用的是 Qt 5.13 或更低版本,可以尝试添加以下头文件:
```cpp
#include <QTextCodec>
```
如果仍然出现找不到文件的错误,请确保你的 Qt 版本与代码中使用的版本匹配,并检查 Qt 安装是否完整。
相关推荐
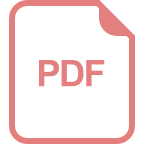














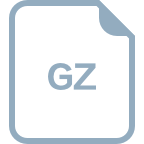