C#使用Action委托编写一段批量读100个int属性和批量写100个int属性的代码,其中属性名分别为MyProperty1~MyProperty100
时间: 2024-10-20 08:06:06 浏览: 19
在C#中,我们可以使用Action委托和反射来编写批量读取和写入100个名为`MyProperty1`到`MyProperty100`的整型属性的代码。首先,假设我们有一个类`ExampleClass`,它有这些属性:
```csharp
public class ExampleClass
{
public int MyProperty1 { get; set; }
// ... 重复99次...
public int MyProperty100 { get; set; }
}
```
然后,我们可以创建两个Action委托,分别用于读取和写入属性值:
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
using System.Reflection;
public static void BatchReadAndWriteProperties(IEnumerable<ExampleClass> objects)
{
Action<ExampleClass, PropertyInfo> readAction = (obj, prop) => Console.WriteLine($"Reading property '{prop.Name}': {prop.GetValue(obj)}");
Action<ExampleClass, string, int> writeAction = (obj, propertyName, value) =>
{
PropertyInfo property = obj.GetType().GetProperty(propertyName);
if (property != null)
property.SetValue(obj, value);
};
List<string> propertyNames = new List<string> { "MyProperty1", "MyProperty2", ..., "MyProperty100" }; // 生成实际的属性名称列表
foreach (var objectInstance in objects)
{
foreach (string propertyName in propertyNames)
{
readAction(objectInstance, objectInstance.GetType().GetProperty(propertyName));
// 这里你可以添加具体的值来写入,例如:
// writeAction(objectInstance, propertyName, 42); // 替换为实际想要的值
}
}
}
// 示例用法:
List<ExampleClass> exampleObjects = new List<ExampleClass>(); // 创建并填充exampleObjects
BatchReadAndWriteProperties(exampleObjects);
```
请注意,在实际应用中,批处理操作可能会涉及数据库、文件或其他复杂的数据源,这里示例代码仅用于演示如何读取和设置属性。
阅读全文
相关推荐
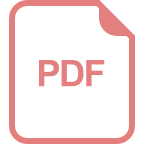
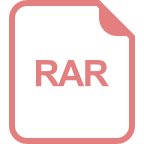
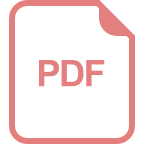















