python推箱子游戏源代码
时间: 2023-12-07 15:38:41 浏览: 109
很高兴为您介绍Python推箱子游戏的源代码。由于推箱子游戏的实现方式有很多种,我将为您提供一种基于Python的实现方式。
```python
# 定义地图类
class Map:
def __init__(self, map_list):
self.map_list = map_list
self.row = len(map_list)
self.col = len(map_list[0])
self.player_pos = None
self.box_pos = []
self.target_pos = []
for i in range(self.row):
for j in range(self.col):
if map_list[i][j] == 'P':
self.player_pos = (i, j)
elif map_list[i][j] == 'B':
self.box_pos.append((i, j))
elif map_list[i][j] == 'T':
self.target_pos.append((i, j))
# 判断是否胜利
def is_win(self):
for pos in self.box_pos:
if pos not in self.target_pos:
return False
return True
# 移动箱子
def move_box(self, box_pos, direction):
new_box_pos = (box_pos[0] + direction[0], box_pos[1] + direction[1])
if self.map_list[new_box_pos[0]][new_box_pos[1]] == '#':
return False
if new_box_pos in self.box_pos:
return False
self.box_pos.remove(box_pos)
self.box_pos.append(new_box_pos)
return True
# 移动玩家
def move_player(self, direction):
new_player_pos = (self.player_pos[0] + direction[0], self.player_pos[1] + direction[1])
if self.map_list[new_player_pos[0]][new_player_pos[1]] == '#':
return False
for box_pos in self.box_pos:
if new_player_pos == box_pos:
if not self.move_box(box_pos, direction):
return False
break
self.player_pos = new_player_pos
return True
# 打印地图
def print_map(self):
for i in range(self.row):
for j in range(self.col):
if (i, j) == self.player_pos:
print('P', end='')
elif (i, j) in self.box_pos:
print('B', end='')
elif (i, j) in self.target_pos:
print('T', end='')
elif self.map_list[i][j] == '#':
print('#', end='')
else:
print(' ', end='')
print()
# 定义主函数
def main():
map_list = [
['#', '#', '#', '#', '#', '#', '#', '#', '#', '#'],
['#', ' ', ' ', ' ', '#', ' ', ' ', ' ', ' ', '#'],
['#', ' ', ' ', ' ', '#', ' ', ' ', ' ', ' ', '#'],
['#', ' ', ' ', ' ', ' ', ' ', 'B', ' ', ' ', '#'],
['#', ' ', ' ', ' ', ' ', ' ', '#', ' ', ' ', '#'],
['#', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', '#'],
['#', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', '#'],
['#', ' ', ' ', ' ', ' ', ' ', ' ', 'T', ' ', '#'],
['#', ' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ', '#'],
['#', '#', '#', '#', '#', '#', '#', '#', '#', '#']
]
game_map = Map(map_list)
game_map.print_map()
while not game_map.is_win():
direction = input('请输入移动方向(w:上 s:下 a:左 d:右):')
if direction == 'w':
if not game_map.move_player((-1, 0)):
print('移动失败,请重新输入')
elif direction == 's':
if not game_map.move_player((1, 0)):
print('移动失败,请重新输入')
elif direction == 'a':
if not game_map.move_player((0, -1)):
print('移动失败,请重新输入')
elif direction == 'd':
if not game_map.move_player((0, 1)):
print('移动失败,请重新输入')
else:
print('输入有误,请重新输入')
game_map.print_map()
print('恭喜你,游戏胜利!')
if __name__ == '__main__':
main()
```
阅读全文
相关推荐

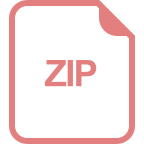
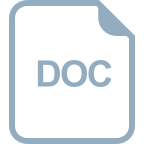
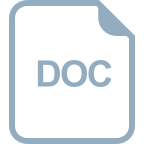
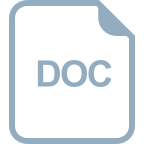



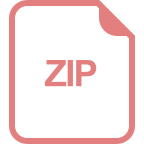
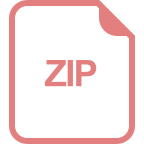
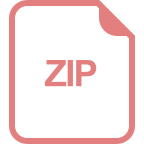
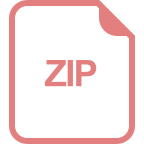
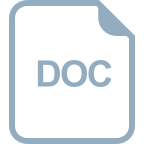
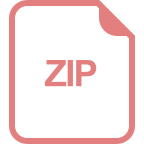