由键盘任意输入两串长度最大为1024字符的字符串,不用库函数strcat,连接两串字符串,用C语言代码
时间: 2024-11-12 15:37:57 浏览: 21
在C语言中,如果不使用`strcat`函数直接连接两个字符串,你可以手动创建一个新的字符串并逐字符添加。这里是一个简单的示例:
```c
#include <stdio.h>
#include <string.h>
// 功能:手动连接两个字符串
void manual_concat(char *result, const char *str1, const char *str2) {
int len1 = strlen(str1);
int len2 = strlen(str2);
// 检查总长度不超过1024字节
if (len1 + len2 + 1 <= 1024) {
result[0] = '\0'; // 初始化结果字符串为空
// 将第一个字符串复制到结果
for (int i = 0; i < len1; i++) {
result[i + 1] = str1[i]; // 结果字符串后加一位置用于存储字符
}
result[len1] = '\0'; // 添加第一个字符串结束符
// 将第二个字符串追加到结果
for (int j = 0; j < len2; j++) {
result[len1 + 1 + j] = str2[j];
}
result[len1 + len2] = '\0'; // 添加第二个字符串结束符
} else {
printf("字符串长度超过限制,无法连接!\n");
}
}
int main() {
char str1[1025], str2[1025], result[1025];
// 输入字符串
fgets(str1, sizeof(str1), stdin); // 去除换行符
fgets(str2, sizeof(str2), stdin);
manual_concat(result, str1, str2);
printf("连接后的字符串: %s\n", result);
return 0;
}
```
在这个例子中,我们首先计算了两个输入字符串的长度,然后检查它们的总长度是否在1024字符以内。接着手动将每个字符从一个字符串复制到新创建的结果字符串中,并在合适的位置添加结束符`\0`。
阅读全文
相关推荐
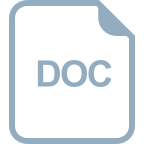
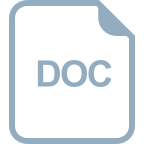
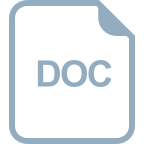
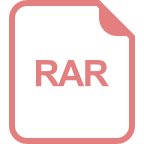
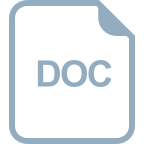
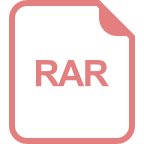
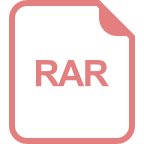











