练习:练习使用Pvthon实现HTTP请求的三种方式(urllib模块、urllib3模块、requests模块)每种方式采用get和post两种请求模式,给出代码运行情况截图。
时间: 2024-09-15 15:01:49 浏览: 42
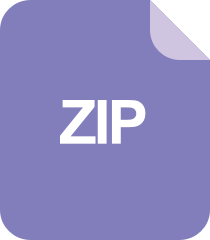
毕业设计:Python基于深度学习的聊天机器人的设计与实现(源码 + 数据库 + 说明文档)

Python中实现HTTP请求有多种库可以选择,其中常见的有`urllib`模块,`urllib3`库(它是`urllib`的一个增强版),以及第三方库`requests`。以下是使用这三种方式进行GET和POST请求的基本示例:
1. 使用`urllib`模块:
```python
import urllib.request
# GET请求
response_get = urllib.request.urlopen('http://example.com')
data_get = response_get.read().decode()
print(data_get)
# POST请求 (需要数据编码)
data = 'key=value&another_key=another_value'
headers = {'Content-Type': 'application/x-www-form-urlencoded'}
req = urllib.request.Request('http://example.com', data=data.encode(), headers=headers)
with urllib.request.urlopen(req) as response_post:
data_post = response_post.read().decode()
print(data_post)
```
2. 使用`urllib3`模块:
```python
import urllib3
# 创建一个池化管理器
http = urllib3.PoolManager()
# GET请求
response_get = http.request('GET', 'http://example.com')
print(response_get.data.decode())
# POST请求
response_post = http.request('POST', 'http://example.com', fields={'key': 'value'})
print(response_post.data.decode())
```
3. 使用`requests`库:
```python
import requests
# GET请求
response_get = requests.get('http://example.com')
print(response_get.text)
# POST请求
response_post = requests.post('http://example.com', data={'key': 'value'})
print(response_post.text)
```
由于这里无法提供代码运行截图,建议你在本地尝试运行以上代码,并查看控制台输出的结果。在实际环境中,确保网络连接并替换URL为实际可用的服务地址。
阅读全文
相关推荐
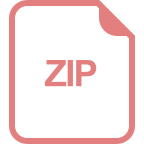
















