用工厂方法模式实现洗衣机工厂的功能类图
时间: 2024-10-19 09:06:39 浏览: 33
工厂方法模式是一种创建型设计模式,它提供了一个用于创建对象的抽象工厂,而无需指定具体的产品类。在这个场景下,如果你要实现一个洗衣机工厂的功能,可以构建如下的类图:
1. **抽象洗衣机接口** (WashingMachine): 定义所有洗衣机共有的功能,如洗涤、漂洗、烘干等。
```java
public interface WashingMachine {
void wash();
void rinse();
void dry();
}
```
2. **基础洗衣机类** (BaseWasher): 提供基本的洗衣机功能,并作为其他具体洗衣机的基类。
```java
public abstract class BaseWasher implements WashingMachine {
// 具体的洗涤、漂洗和烘干方法实现
}
```
3. **波轮洗衣机类** (SpinDrierWasher): 实现基于波轮的工作原理。
```java
public class SpinDryerWasher extends BaseWasher {
// 波轮洗衣机特有的实现
}
```
4. **滚筒洗衣机类** (RollerWasher): 另一种洗衣机类型的实现。
```java
public class RollerWasher extends BaseWasher {
// 滚筒洗衣机特有的实现
}
```
5. **洗衣机工厂类** (WashingMachineFactory): 创建并返回相应类型的洗衣机实例。
```java
public class WashingMachineFactory {
public static WashingMachine createWashingMachine(String type) {
if ("spin_drier".equals(type)) {
return new SpinDrierWasher();
} else if ("roller".equals(type)) {
return new RollerWasher();
} else {
throw new IllegalArgumentException("Invalid washing machine type");
}
}
}
```
阅读全文
相关推荐
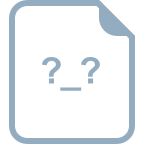
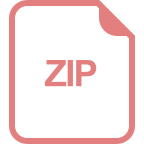











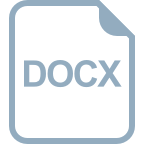
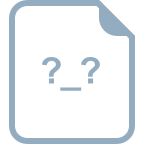
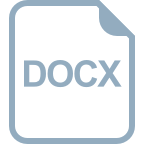

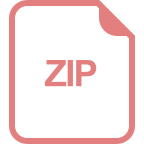