JSONObject 排序
时间: 2023-12-02 18:08:44 浏览: 37
要对JSONObject进行排序,需要先将其转换为可以排序的数据结构,比如Map或List。然后根据你想要的排序规则对数据结构进行排序,最后再将排序后的结果转换回JSONObject。
下面是一个示例代码,演示了如何对JSONObject进行排序:
```java
import org.json.JSONObject;
import java.util.*;
public class JSONObjectSorting {
public static void main(String[] args) {
JSONObject jsonObject = new JSONObject();
jsonObject.put("name", "John");
jsonObject.put("age", 25);
jsonObject.put("city", "New York");
// 将JSONObject转换为Map
Map<String, Object> map = new HashMap<>();
Iterator<String> keys = jsonObject.keys();
while (keys.hasNext()) {
String key = keys.next();
Object value = jsonObject.get(key);
map.put(key, value);
}
// 将Map按键排序
Map<String, Object> sortedMap = new TreeMap<>(map);
// 将排序后的Map转换回JSONObject
JSONObject sortedJsonObject = new JSONObject(sortedMap);
System.out.println(sortedJsonObject.toString());
}
}
```
在这个例子中,我们首先将JSONObject转换为一个Map,然后使用TreeMap对Map进行排序。最后将排序后的Map再转换回JSONObject。通过这种方式,你可以得到一个按键排序后的JSONObject。
注意:JSONObject是无序的数据结构,所以在转换为其他数据结构进行排序时,可能会丢失原有的顺序。
相关推荐
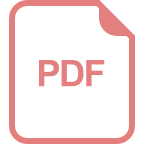
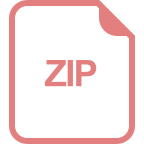
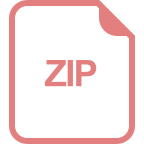













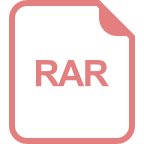