小蓝鲸们有一个庞大的家族,家族成员间的亲属关系可以构成一棵二叉树。在小蓝鲸家族的族谱中记录了两个整数数组preorder和inorder, 其中preorder是二叉树的先序遍历,inorder是这棵树的中序遍历,请构造二叉树,并找到亲属关系最远的两个小蓝鲸之间的距离。
时间: 2024-11-11 22:22:07 浏览: 6
在计算机科学中,给定先序遍历(preorder)和中序遍历(inorder)重建一颗二叉搜索树(BST),然后寻找其中两个节点之间的最长路径(即“亲属关系最远”),通常涉及以下几个步骤:
1. **重构树结构**:先序遍历的第一个元素是根节点,接着用中序遍历找到根节点的位置,分割出左子树的中序遍历和右子树的中序遍历。分别对左右子树递归地做同样的过程。
2. **构建BST**:通过上述信息,我们可以创建一个二叉树的数据结构,每个节点包含值、左子节点指针和右子节点指针。
3. **查找最远路径**:对于这个问题,我们通常会采用层次遍历(广度优先搜索)的方式,计算从每个节点到其所有后代中最远节点的距离。在这个过程中,我们会维护一个队列,存储每个节点及其到达距离。最后,找出距离差最大的两对节点。
4. **找出最长路径**:遍历完成后,最长路径即为两个节点的距离差,这两个节点可能是兄弟节点或者是祖孙节点等。
要解决这个问题,你需要编写一个算法来处理输入数据并计算最长路径。如果你需要具体的代码实现,我可以提供指导,但完整的代码将取决于使用的编程语言。以下是Python的一个简单伪代码示例:
```python
def build_tree(preorder, inorder):
# 先序遍历的第一个元素是根
root_value = preorder[0]
# 找到根在中序遍历中的位置
index = inorder.index(root_value)
# 递归创建左右子树
left_tree = build_tree(preorder[1:index+1], inorder[:index])
right_tree = build_tree(preorder[index+1:], inorder[index+1:])
return TreeNode(root_value, left_tree, right_tree)
# 完成树的构建后,使用层次遍历找最远路径
def find_longest_path_distance(tree):
queue = [(tree, 0)]
max_distance = 0
path1, path2 = None, None
while queue:
node, distance = queue.pop(0)
if not node.left and not node.right:
# 如果是最远节点,更新路径和最大距离
if path1 is None or (path2 is not None and distance - path1[1] > max_distance):
path2 = path1
max_distance = distance - path1[1]
elif path2 is None or distance - path2[1] > max_distance:
path2 = (node, distance)
if path1 is None or distance - path1[1] > max_distance:
path1 = (node, distance)
if node.left:
queue.append((node.left, distance + 1))
if node.right:
queue.append((node.right, distance + 1))
return max_distance, (path1, path2)
# 输入preorder和inorder,应用函数获取结果
max_distance, furthest_nodes = find_longest_path_distance(build_tree(preorder, inorder))
```
阅读全文
相关推荐
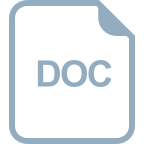
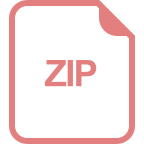
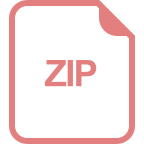
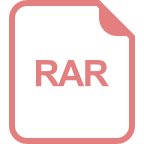
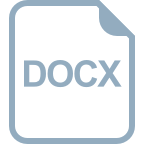
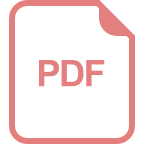
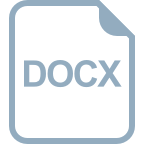
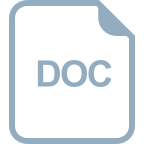
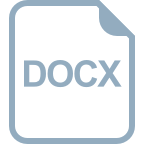
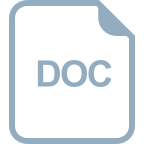
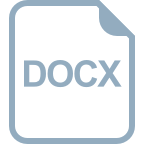
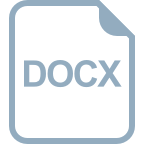
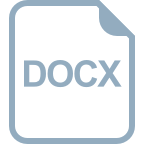
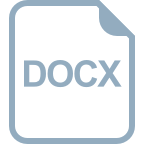
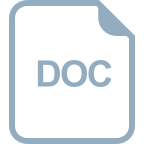

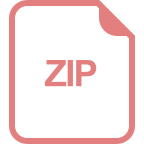