完善代码使其能正常运行
时间: 2024-09-07 12:03:27 浏览: 37
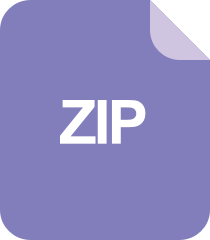
js代码-封装完善自己的JS代码库
为了使提供的作物种植计划优化代码能够正确执行,我们需要解决几个关键点:
- 首先确认所有必要的库已经导入,并且在实际使用前定义了所需的变量。
- 其次要保证`a`, `revenue`, 和`max_area`这些矩阵或向量包含正确的值并且它们的数据结构符合预期。
- 此外还需确保模型中的变量名称和访问方式一致无误。
- 最后,由于示例中缺少具体数值,我们将用占位符来表示应该插入真实数据的地方。
以下是调整后的代码:
```python
import pulp
import pandas as pd
# 初始化模型
model = pulp.LpProblem("Crop Planting Plan", pulp.LpMaximize)
# 定义变量
crops = list(range(0, 22))
plots = [
'A1', 'A2', 'A3', 'A4', 'A5', 'A6',
*[f'B{n}' for n in range(1, 15)],
*[f'C{n}' for n in range(1, 7)],
*[f'D{n}' for n in range(1, 9)],
*[f'E{n}' for n in range(1, 17)],
*[f'F{n}' for n in range(1, 5)]
]
crop_plots = [(plot, crop) for plot in plots for crop in crops]
# 声明决策变量
x = pulp.LpVariable.dicts("Crop_planting", (plots, crops), lowBound=0, cat='Continuous')
# 填充收益系数矩阵 a (此处应替换为实际数据)
a = [[... for _ in range(len(crops))] for _ in range(len(plots))]
# 收益表 revenue (同样需要替换成实际收益数据)
revenue = [[... for _ in range(len(crops))] for _ in range(len(plots))]
# 目标函数:最大化总收入
model += pulp.lpSum(revenue[plot][crop] * x[plot][crop] * a[plot][crop] for plot, crop in crop_plots)
# 每个地块的最大可利用面积列表(请根据实际情况填写)
max_area = [
365, 365, 365, 365, 365, 365,
619]*12 + [108]*6 + [109]*6 + [109]*6 + [9.6]*18 + [2.4]*4
assert len(max_area) == len(plots), "max_area 的长度应等于 plots"
# 添加约束条件
for idx, plot in enumerate(plots):
model += (
pulp.lpSum(x[plot][crop] for crop in crops) <= max_area[idx],
f"AreaLimit_{plot}"
)
# 解决模型
status = model.solve()
print(f'Status: {pulp.LpStatus[status]}')
if status == pulp.LpStatusOptimal:
# 输出结果
results = []
for variable in model.variables():
if variable.varValue > 0:
result = {
'Plot': int(variable.name.split('_')[1]),
'Crop': int(variable.name.split('_')[2].split('.')[0]), # 处理浮点数索引情况
'Quantity': variable.varValue,
}
results.append(result)
output_df = pd.DataFrame(results)
print(output_df.head())
else:
print('No optimal solution found.')
```
请注意,在上述代码中,“…”代表你需要填入的实际数值或者从文件读取的数组。同时,对于决策变量的名字解析部分,我做了细微修改以处理可能因为浮点数导致的问题。此外,代码中的一些固定数值如地块的最大面积等,你应该依据具体情况予以更新。
阅读全文
相关推荐
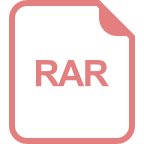
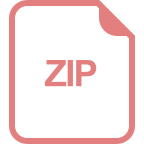





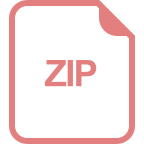
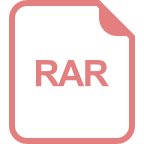
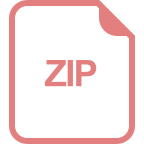
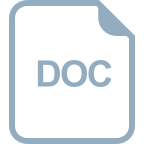

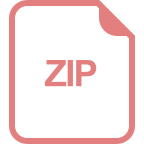
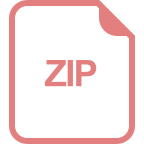