python实现卡尺工具直线检测代码
时间: 2024-12-13 19:13:09 浏览: 10
Python中实现卡尺工具的直线检测通常会用到图像处理库如OpenCV。这里提供一个基本的示例,使用霍夫变换来检测直线:
```python
import cv2
import numpy as np
def find_lines(image):
# 转换为灰度图像并二值化
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
_, binary = cv2.threshold(gray, 50, 255, cv2.THRESH_BINARY_INV)
# 预滤波去除噪声
kernel = cv2.getStructuringElement(cv2.MORPH_RECT, (3, 3))
binary = cv2.morphologyEx(binary, cv2.MORPH_OPEN, kernel)
# 应用霍夫线变换
rho = 1, # 参数设置,描述直线的精度
theta = np.pi/180, # 角度范围,这里是90度
threshold = 15, # 线段长度阈值
min_line_len = 50, # 最小线段长度
max_line_gap = 5, # 线段间最大间隙
lines = cv2.HoughLinesP(binary, rho, theta, threshold, np.array([]), minLineLength=min_line_len, maxLineGap=max_line_gap)
# 根据检测结果画出直线
if lines is not None:
for line in lines:
x1, y1, x2, y2 = line[0]
cv2.line(image, (x1, y1), (x2, y2), (0, 255, 0), 2) # 绿色线条
return image
# 加载图像
image = cv2.imread('your_image_path.jpg')
result = find_lines(image)
cv2.imshow('Linear Detection', result)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这个代码首先将图像转换为灰度,并进行一些预处理步骤以增强直线的对比度。然后,它应用霍夫线变换来寻找直线,最后在原始图像上绘制出来。
阅读全文
相关推荐
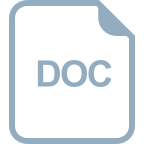
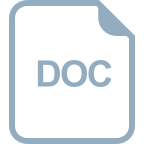
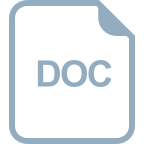
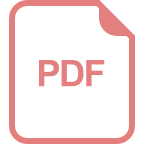
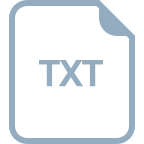
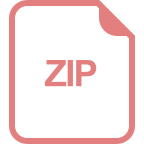
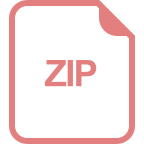
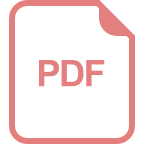
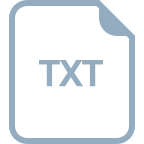
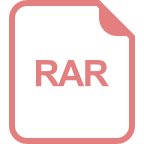
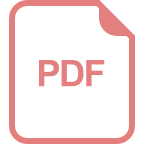
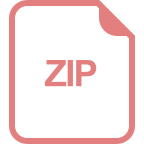
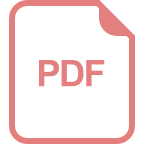