为线性表类添加一个方法,其功能为删除第i号位置开始的k个元素
时间: 2024-09-19 16:17:57 浏览: 25
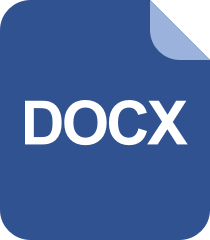
数据结构课后习题答案第二章 线性表.docx
在创建线性表(如数组、链表等)类时,如果你想添加一个方法来删除从指定位置开始的k个元素,这个操作通常涉及到对数据结构的具体实现有特定的要求。这里以链表为例来说明:
```java
// 假设我们有一个Node类代表链表节点,包含一个值val和指向下一个节点的引用next
public class LinkedList {
private Node head;
// 其他链表操作...
/**
* 删除从第i号位置开始的k个元素
* @param i 起始位置索引(0-based)
* @param k 需要删除的元素数量
*/
public void deleteElements(int i, int k) {
if (i < 0 || i >= size() || k <= 0) {
throw new IllegalArgumentException("Invalid input: position and count must be non-negative and within the list bounds.");
}
if (i == 0 && k > 1) { // 如果要删除的是头结点,特殊处理
for (int j = 0; j < k - 1; j++) {
head = head.next;
}
} else { // 普通情况,遍历删除
Node current = head;
Node previous = null;
for (int j = 0; j < k; j++) {
if (j >= i) { // 进入需要删除的部分
if (previous != null) {
previous.next = current.next; // 更新前一个节点的next指针
} else {
head = current.next; // 如果删除第一个元素,head也要更新
}
current = current.next; // 移动到下一个节点
} else { // 未到达删除区域,继续向前查找
previous = current;
current = current.next;
}
}
}
}
// 线程相关的辅助方法...
}
```
阅读全文
相关推荐
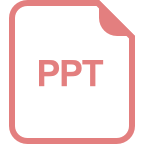
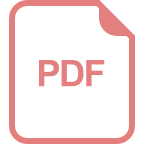

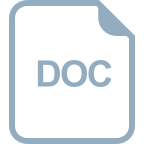
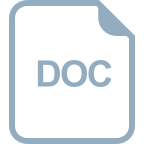
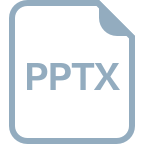
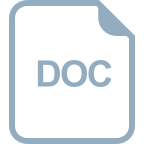
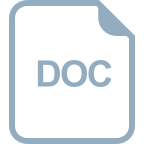
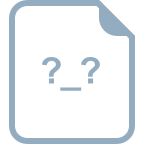
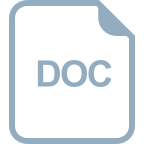
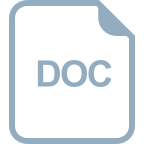
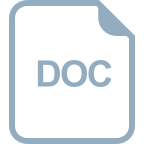





