python人民币大写
时间: 2024-08-31 19:00:35 浏览: 26
Python中可以使用第三方库`moneyed`或者自定义函数来将人民币金额转换成大写的形式。例如,`moneyed`库提供了一个方便的方式来处理货币格式和大写转换。下面是一个简单的例子:
```python
from moneyed import CNY
def cny_to_big_str(amount):
currency = CNY(0) # 初始化人民币
return format(currency(amount), 'Chinese') # 使用内置的format函数, 格式化为中国大写
# 示例
amount = 12345.67
big_amount = cny_to_big_str(amount)
print(f"人民币{amount}元的大写形式为: {big_amount}")
```
如果你不想使用外部库,也可以通过字符串替换的方式实现,但可能会复杂一些,需要处理数字和单位的对应关系。
相关问题
Python人民币大小写转换
Python中可以使用第三方库`rmbconvert`来实现人民币大小写转换。可以使用pip安装该库:`pip install rmbconvert`。下面是一个简单的示例:
```
from rmbconvert import RMBConvert
rmb = RMBConvert()
print(rmb.to_rmb_upper(1234567890.12)) # 壹拾贰亿叁仟肆佰伍拾陆万柒仟捌佰玖拾元壹角贰分
```
以上代码使用了`RMBConvert`类中的`to_rmb_upper`方法将数字金额转换为人民币大写形式。
python实现数字转人民币
在Python中,你可以使用一些字符串格式化的方法来将数字转换成人民币大写形式。以下是一个简单的示例,它使用了`format`函数和预定义的货币单位:
```python
def num_to_rmb(num):
# 定义货币单位
unit = ['', '元', '角', '分']
# 将数值转换为整数并取整
num = int(round(num))
# 计算每一位对应的货币单位
rmb_str = ''
while num > 0:
digit = num % 10
rmb_str = unit[digit] + rmb_str
num = num // 10
# 添加"人民币"前缀
rmb_str = "人民币{}{}".format(''.join(rmb_str[::-1]), '整' if num == 0 else '')
return rmb_str
# 示例
amount = 123.456
print(num_to_rmb(amount)) # 输出:人民币壹佰贰拾叁圆肆角陆分
```
这个函数会处理小数部分,并按照中国的传统习惯,如“一元”、“两角”等。
相关推荐
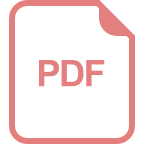
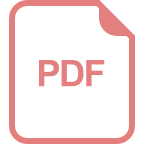
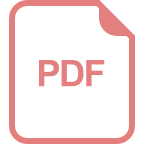












