在Linux中编写程序,在程序中创建一个子进程,使子进程通过exec更改代码段,执行cat命令。并运行该程序
时间: 2024-12-10 12:56:53 浏览: 8
在Linux中,你可以使用`fork()`函数创建一个新的子进程,然后使用`exec()`系列函数(如`execvp()`或`execlp()`)替换子进程的当前执行环境,包括代码段。这里是一个简单的C语言示例:
```c
#include <stdio.h>
#include <unistd.h>
#include <stdlib.h>
#include <string.h>
int main() {
// 创建子进程
pid_t child_pid = fork();
if (child_pid == -1) { // fork失败,打印错误信息并退出
perror("Fork failed");
return 1;
} else if (child_pid == 0) { // 子进程
char *cmd[] = {"cat", "/path/to/your/file.txt", NULL}; // 替换为你要执行的文件路径
execvp(cmd[0], cmd); // 使用execvp执行指定的命令
perror("Execvp failed");
exit(1);
} else { // 父进程
printf("Parent process: Child process ID is %d\n", child_pid);
wait(NULL); // 等待子进程结束
}
return 0;
}
```
在这个程序中,`fork()`会返回0给子进程,非零值给父进程。子进程中,我们指定了`"cat"`命令和文件路径,并用`execvp()`替换它。如果一切顺利,子进程将执行`cat`命令,而父进程则等待子进程结束。
阅读全文
相关推荐
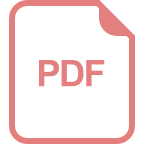
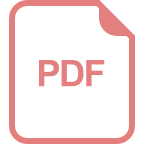
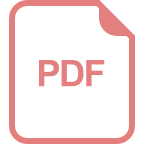


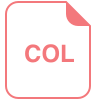
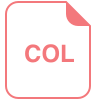
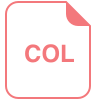
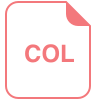
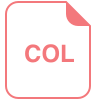
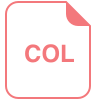
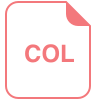
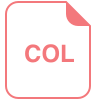
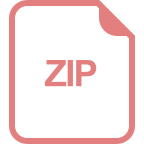
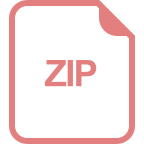