list用stream排序
时间: 2024-01-26 18:12:36 浏览: 23
在Java中,可以使用Stream API对List进行排序。具体操作如下:
1. 首先,需要导入以下包:
```java
import java.util.Comparator;
import java.util.List;
import java.util.stream.Collectors;
```
2. 假设有一个自定义类`Person`,包含`age`和`name`两个属性,我们需要根据`age`对`List<Person>`进行排序:
```java
class Person {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
```
3. 使用Stream API对List进行排序:
```java
List<Person> people = new ArrayList<>();
people.add(new Person("张三", 30));
people.add(new Person("李四", 25));
people.add(new Person("王五", 35));
List<Person> sortedPeople = people.stream()
.sorted(Comparator.comparingInt(Person::getAge))
.collect(Collectors.toList());
```
在这个例子中,我们使用了`Comparator.comparingInt()`方法根据`Person`对象的`age`属性进行排序。最后,使用`collect()`方法将排序后的Stream转换回List。
相关推荐
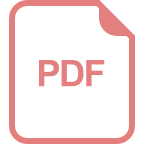
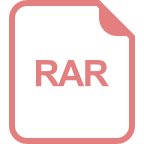
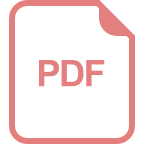














