C++使用CString变量
时间: 2024-09-08 09:01:43 浏览: 51
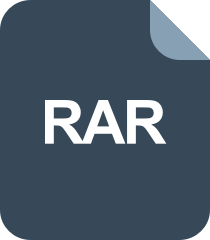
C++ Cstring的源码
CString是MFC(Microsoft Foundation Classes)库中定义的一个非常方便的字符串类,它提供了一系列方法来处理字符串,比如字符串的连接、比较、查找等。CString类封装了C风格字符串(char*)的复杂操作,使得字符串操作更为简单和安全。
使用CString变量时,你不需要手动管理内存分配和释放,因为CString内部会自动处理。你可以像使用普通数据类型一样直接赋值、连接等。此外,CString还能够自动处理Unicode和多字节字符集之间的转换。
下面是一个简单的示例,展示了如何在C++中使用CString:
```cpp
#include <afx.h> // 包含MFC核心组件
int main() {
// 创建CString对象
CString strHello("Hello, World!");
// 使用+操作符连接CString对象
strHello = strHello + " This is a test.";
// 使用+=操作符追加字符串
strHello += " Welcome to C++ programming.";
// 输出CString对象的内容
AfxMessageBox(strHello);
return 0;
}
```
阅读全文
相关推荐
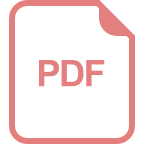
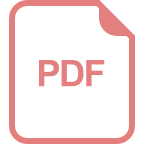















