md5 c++语言实现
时间: 2023-11-14 11:02:12 浏览: 48
MD5是一种广泛使用的散列算法,用于确保信息在传输过程中的完整性和一致性。MD5算法可以将任意长度的数据运算转化为一个固定长度的摘要。在C语言中,可以使用MD5算法来实现文件的完整性校验。
在Linux平台上,可以使用C语言编写一个基于MD5算法的文件完整性校验程序。该程序可以通过命令行参数进行不同的操作。例如,使用`./md5 -h`可以查看程序的帮助信息,使用`./md5 -t`可以打印程序的测试信息,使用`./md5 -c nankai.txt`可以计算并打印被测文件的MD5摘要。
下面是一个示例的C语言代码,用于实现MD5算法的文件完整性校验程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/md5.h>
void calculate_md5(const char* filename) {
FILE* file = fopen(filename, "rb");
if (file == NULL) {
printf("Unable to open file: %s\n", filename);
return;
}
MD5_CTX context;
MD5_Init(&context);
unsigned char buffer[1024];
int bytesRead;
while ((bytesRead = fread(buffer, 1, sizeof(buffer), file)) != 0) {
MD5_Update(&context, buffer, bytesRead);
}
unsigned char digest[MD5_DIGEST_LENGTH];
MD5_Final(digest, &context);
fclose(file);
printf("MD5 digest of file '%s': ", filename);
for (int i = 0; i < MD5_DIGEST_LENGTH; i++) {
printf("%02x", digest[i]);
}
printf("\n");
}
int main(int argc, char* argv[]) {
if (argc != 3) {
printf("Usage: %s -c <filename>\n", argv[0]);
return 1;
}
if (strcmp(argv[1], "-c") == 0) {
calculate_md5(argv[2]);
} else {
printf("Invalid option: %s\n", argv[1]);
}
return 0;
}
```
这个示例程序使用了OpenSSL库中的MD5相关函数来计算文件的MD5摘要。它通过打开文件、逐块读取数据并进行摘要计算,最后输出计算得到的摘要结果。
相关推荐
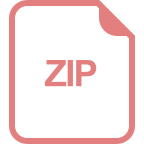
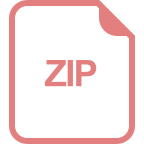














