python 凯撒密码栅栏密码
时间: 2023-10-25 18:04:50 浏览: 150
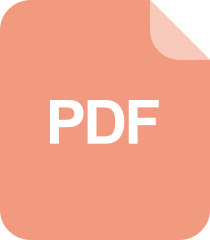
利用python实现凯撒密码加解密
Python可以使用以下方式实现凯撒密码和栅栏密码:
凯撒密码是一种简单的密码转换方法,通过对原文中的每个字母进行移位来加密和解密。在Python中,可以使用ord()和chr()函数来实现字母的ASCII码和字符之间的转换。
例如,我们要将明文字符串"HELLO"使用凯撒密码进行加密,假设移位值为3:
```python
def caesar_cipher_encrypt(plaintext, shift):
ciphertext = ""
for char in plaintext:
if char.isalpha(): # 只对字母进行加密
ascii_value = ord(char.upper()) # 转换为大写字母的ASCII码
shifted_ascii_value = (ascii_value - 65 + shift) % 26 + 65 # 字母移位
ciphertext += chr(shifted_ascii_value) # 转换为加密后的字符
else:
ciphertext += char # 非字母字符直接添加到密文中
return ciphertext
plaintext = "HELLO"
shift = 3
ciphertext = caesar_cipher_encrypt(plaintext, shift)
print(ciphertext) # 输出:KHOOR
```
栅栏密码是一种换位密码,通过将明文的字母按照一定的规则重新排列来加密和解密。在Python中,可以使用循环和切片操作来实现栅栏密码。
例如,我们要将明文字符串"HELLO"使用栅栏密码进行加密,假设栏数为2:
```python
def rail_fence_cipher_encrypt(plaintext, num_rails):
rails = [[] for _ in range(num_rails)] # 创建栅栏列表
current_rail = 0
direction = 1 # 标识方向
for char in plaintext:
rails[current_rail].append(char) # 根据当前栏添加字符
if current_rail == num_rails - 1: # 到达最后一栏时改变方向
direction = -1
elif current_rail == 0: # 回到第一栏时改变方向
direction = 1
current_rail += direction # 改变当前栏
ciphertext = ""
for rail in rails:
ciphertext += "".join(rail) # 将栏内字符拼接成密文
return ciphertext
plaintext = "HELLO"
num_rails = 2
ciphertext = rail_fence_cipher_encrypt(plaintext, num_rails)
print(ciphertext) # 输出:HLOEL
```
以上就是使用Python实现凯撒密码和栅栏密码的简单示例,这些代码可以作为基础来进一步扩展和优化。
阅读全文
相关推荐
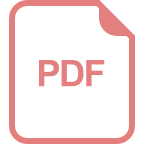
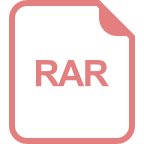
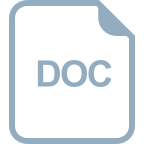
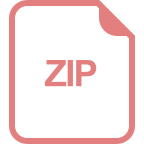
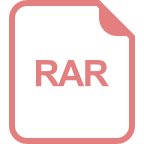
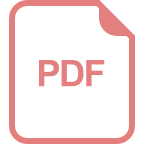
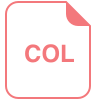
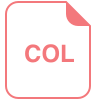
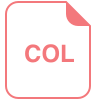
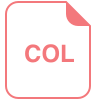
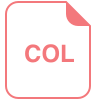
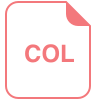
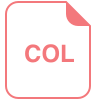
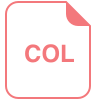
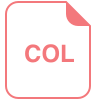
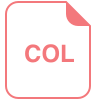
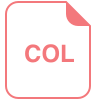