1、实现查询二叉树中的某个结点的算法2、实现统计二叉树中叶子结点个数的算法3、实现统计二叉树中所有结点个数的算法4、实现求二叉树深度的算法
时间: 2024-12-16 13:23:55 浏览: 6
1. 查找二叉树中的特定节点通常使用递归的方式,如“先序遍历”或“中序遍历”。首先检查根节点是否就是目标节点,如果不是,则根据目标值与当前节点值的大小关系,分别在左子树或右子树中继续搜索。递归直到找到目标节点或遍历到空树。伪代码如下:
```python
def search_binary_tree(root, target):
if root is None or root.val == target:
return root
elif target < root.val:
return search_binary_tree(root.left, target)
else:
return search_binary_tree(root.right, target)
```
2. 统计二叉树中叶子节点的算法也很简单,可以在遍历过程中计数。因为叶子节点既没有左孩子也没有右孩子,所以判断条件相对直接。以下是Python代码示例:
```python
def count_leaves(node):
if node is None:
return 0
if node.left is None and node.right is None:
return 1
return count_leaves(node.left) + count_leaves(node.right)
```
3. 计算二叉树的所有节点总数,同样可以采用递归遍历,对每个节点都加一。下面是算法实现:
```python
def count_nodes(node):
if node is None:
return 0
return 1 + count_nodes(node.left) + count_nodes(node.right)
```
4. 求解二叉树的深度,可以使用“广度优先搜索”(BFS),从根节点开始逐层遍历,记录每层的最大深度。如果二叉树非空,深度至少为1。伪代码如下:
```python
from collections import deque
def max_depth(root):
if root is None:
return 0
queue = deque([(root, 1)])
while queue:
node, depth = queue.popleft()
if node.left is not None:
queue.append((node.left, depth + 1))
if node.right is not None:
queue.append((node.right, depth + 1))
return depth
```
阅读全文
相关推荐
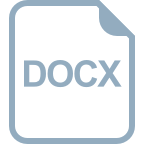
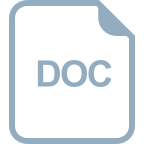
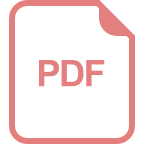
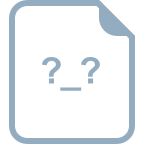
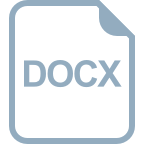
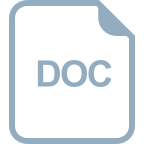
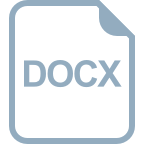
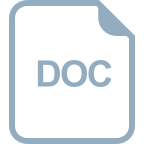
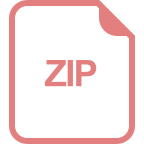
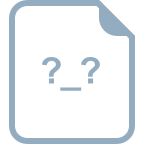
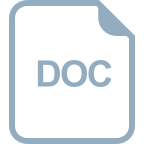
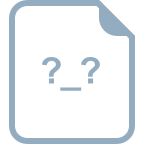
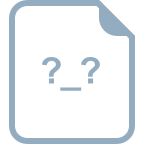
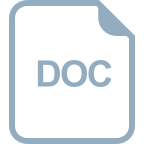
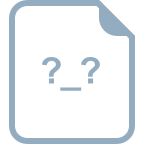
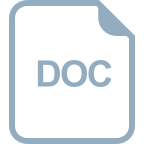
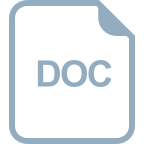