ffmpeg解码jpeg C++
时间: 2024-01-11 07:22:37 浏览: 214
ffmpeg是一个开源的音视频处理工具,可以用于解码和编码各种音视频格式。在C++中使用ffmpeg解码jpeg文件可以按照以下步骤进行:
1. 引入ffmpeg库和头文件:
```cpp
extern "C" {
#include <libavcodec/avcodec.h>
#include <libavformat/avformat.h>
#include <libswscale/swscale.h>
}
```
2. 初始化ffmpeg:
```cpp
av_register_all();
```
3. 打开输入文件:
```cpp
AVFormatContext* formatContext = avformat_alloc_context();
if (avformat_open_input(&formatContext, "input.jpg", NULL, NULL) != 0) {
// 打开文件失败的处理
}
if (avformat_find_stream_info(formatContext, NULL) < 0) {
// 获取流信息失败的处理
}
```
4. 查找视频流:
```cpp
int videoStreamIndex = -1;
for (int i = 0; i < formatContext->nb_streams; i++) {
if (formatContext->streams[i]->codecpar->codec_type == AVMEDIA_TYPE_VIDEO) {
videoStreamIndex = i;
break;
}
}
if (videoStreamIndex == -1) {
// 没有找到视频流的处理
}
```
5. 获取解码器并打开解码器:
```cpp
AVCodecParameters* codecParameters = formatContext->streams[videoStreamIndex]->codecpar;
AVCodec* codec = avcodec_find_decoder(codecParameters->codec_id);
AVCodecContext* codecContext = avcodec_alloc_context3(codec);
if (avcodec_parameters_to_context(codecContext, codecParameters) < 0) {
// 获取解码器上下文失败的处理
}
if (avcodec_open2(codecContext, codec, NULL) < 0) {
// 打开解码器失败的处理
}
```
6. 创建帧对象和缓冲区:
```cpp
AVFrame* frame = av_frame_alloc();
AVPacket* packet = av_packet_alloc();
uint8_t* buffer = (uint8_t*)av_malloc(av_image_get_buffer_size(AV_PIX_FMT_RGB24, codecContext->width, codecContext->height, 1));
av_image_fill_arrays(frame->data, frame->linesize, buffer, AV_PIX_FMT_RGB24, codecContext->width, codecContext->height, 1);
```
7. 解码并保存为图片:
```cpp
while (av_read_frame(formatContext, packet) >= 0) {
if (packet->stream_index == videoStreamIndex) {
avcodec_send_packet(codecContext, packet);
avcodec_receive_frame(codecContext, frame);
// 将frame保存为图片
}
av_packet_unref(packet);
}
```
8. 释放资源:
```cpp
av_frame_free(&frame);
av_packet_free(&packet);
avcodec_free_context(&codecContext);
avformat_close_input(&formatContext);
```
阅读全文
相关推荐









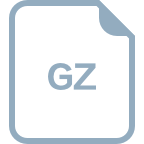

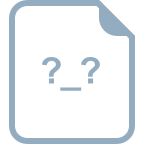






