vue3+ts+vite如何实现网络通信
时间: 2023-09-15 22:23:34 浏览: 102
对于 Vue 3 + TypeScript + Vite 项目,你可以使用 Vue 的官方插件 `vue-axios` 或者 `vue-resource` 来实现网络通信。下面以 `vue-axios` 为例,给出步骤:
1. 安装依赖:
```shell
npm install axios vue-axios
```
2. 在项目的入口文件(一般是 `main.ts`)中引入和使用插件:
```typescript
import { createApp } from 'vue'
import axios from 'axios'
import VueAxios from 'vue-axios'
import App from './App.vue'
const app = createApp(App)
app.use(VueAxios, axios)
app.mount('#app')
```
3. 在需要发送网络请求的组件中使用:
```vue
<template>
<div>
<button @click="fetchData">发送请求</button>
<ul>
<li v-for="item in dataList" :key="item.id">{{ item.text }}</li>
</ul>
</div>
</template>
<script lang="ts">
import { defineComponent } from 'vue'
import { AxiosInstance } from 'axios'
export default defineComponent({
data() {
return {
dataList: []
}
},
methods: {
fetchData() {
// 发送 GET 请求示例
this.axios
.get('/api/data')
.then((response) => {
this.dataList = response.data
})
.catch((error) => {
console.error(error)
})
}
},
mounted() {
// 在 mounted 钩子中,this.axios 可以直接使用
this.fetchData()
}
})
</script>
```
在上述示例中,我们通过 `VueAxios` 插件将 `axios` 实例注入到了 Vue 的实例中,在组件中可以通过 `this.axios` 直接使用。你可以根据接口文档的要求来选择合适的请求方法(例如 `get`、`post`、`put` 等),然后处理返回的结果。
当然,如果你对其他网络请求库或者自己封装的网络请求工具库更感兴趣,也可以在 Vue 3 + TypeScript + Vite 项目中自行选择使用。以上仅供参考。
阅读全文
相关推荐
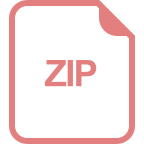
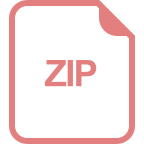
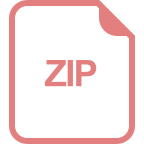
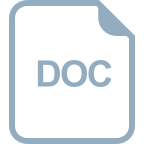
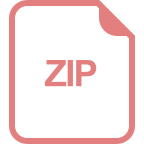
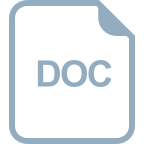
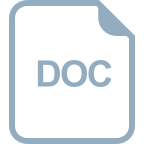

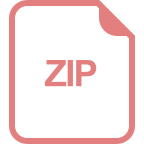
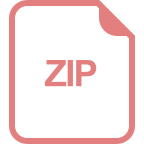
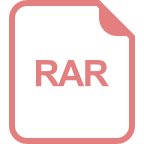
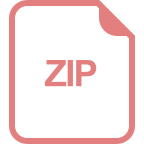
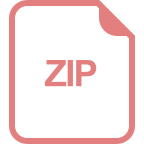
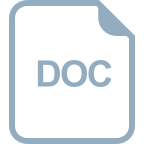
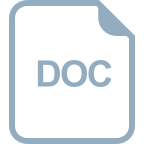
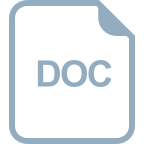
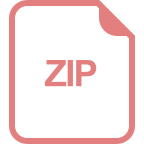