在c#下创建一个基类 Person,包含 Name 和 Age 属性,并定义一个方法 Introduce(),输出 Name 和 Age。
时间: 2024-10-14 09:02:18 浏览: 36
在 C# 中,你可以按照以下步骤创建一个名为 `Person` 的基类,它有 `Name` 和 `Age` 属性,以及一个 `Introduce()` 方法:
```csharp
public class Person
{
// 定义 Name 和 Age 属性
public string Name { get; set; }
public int Age { get; set; }
// 定义 Introduce() 方法,用于输出 Name 和 Age
public virtual void Introduce()
{
Console.WriteLine("Hello, my name is " + Name + " and I am " + Age + " years old.");
}
}
```
在这个基类中,`virtual` 关键字使得 `Introduce()` 方法成为虚方法,这样它的子类可以重写它。当你有一个子类继承自 `Person` 并覆盖了 `Introduce()` 方法,那么该子类就可以根据自身需求提供不同的介绍。
相关问题
创建一个“Person”基类,具有属性“name, age, home”属性,具有“introduceMyself”方法
好的,这是一个简单的Python代码来创建一个“Person”基类,具有“name, age, home”属性,和“introduceMyself”方法:
```python
class Person:
def __init__(self, name, age, home):
self.name = name
self.age = age
self.home = home
def introduceMyself(self):
print(f"My name is {self.name}, I am {self.age} years old and I live in {self.home}.")
```
你可以通过下面的代码来创建一个“Person”对象并调用“introduceMyself”方法来测试:
```python
person = Person("Tom", 25, "New York")
person.introduceMyself()
```
输出应该是:
```
My name is Tom, I am 25 years old and I live in New York.
```
创建一个车辆基类 并包含属性 在创建car和bicyle继承属性 调用相关方法并展示信息
当然,首先我们创建一个简单的`Vehicle`基类,包含通用的属性如`name`和`color`,以及一个抽象的方法`drive()`,表示所有车辆都能行驶。然后我们会为`Car`和`Bicycle`这两个子类提供具体的实现。
```java
// 车辆基类
public abstract class Vehicle {
private String name;
private String color;
// 构造函数
public Vehicle(String name, String color) {
this.name = name;
this.color = color;
}
// 获取属性的方法
public String getName() {
return name;
}
public String getColor() {
return color;
}
// 抽象方法 drive()
public abstract void drive();
// 显示信息的方法
public void displayInfo() {
System.out.println("Name: " + name);
System.out.println("Color: " + color);
System.out.println("This is a vehicle.");
}
}
// Car 子类
public class Car extends Vehicle {
private int numDoors;
public Car(String name, String color, int numDoors) {
super(name, color);
this.numDoors = numDoors;
}
@Override
public void drive() {
System.out.println(getName() + " is driving with " + numDoors + " doors.");
}
}
// Bicycle 子类
public class Bicycle extends Vehicle {
private boolean hasBasket;
public Bicycle(String name, String color, boolean hasBasket) {
super(name, color);
this.hasBasket = hasBasket;
}
@Override
public void drive() {
System.out.println(getName() + " is cycling.");
}
}
```
现在我们可以创建这些类的对象并调用相关方法:
```java
public static void main(String[] args) {
Car car = new Car("Toyota Camry", "Blue", 4);
car.drive(); // 输出: Toyota Camry is driving with 4 doors.
car.displayInfo();
Bicycle bike = new Bicycle("Giant", "Red", true);
bike.drive(); // 输出: Giant is cycling.
bike.displayInfo();
}
```
阅读全文
相关推荐
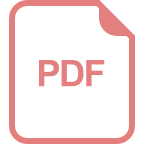
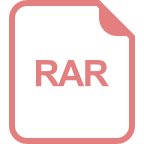
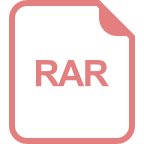













