用python写一份新能源汽车电池管理BMS系统功能设计
时间: 2024-12-15 17:14:51 浏览: 1
新能源汽车电池管理系统(Battery Management System, BMS)是一种关键的车载电子设备,负责监控、保护和优化电池性能。使用Python编写BMS系统的功能设计可以包括以下几个模块:
1. **数据采集**:通过CAN总线或其他通信协议从电池单元获取电压、电流、温度等实时数据,使用Python的socket库或相关的串口库如pyserial来处理通信。
```python
import serial
from can import BusABC
class BatteryDataCollector:
def __init__(self):
self.ser = serial.Serial('COM1', baudrate=9600) # 替换为实际端口
self.bms_bus = BusABC仲裁器(...)
def collect_data(self):
voltage, current, temperature = self.bms_bus.recv_message()
return voltage, current, temperature
```
2. **数据分析与状态监测**:对收集到的数据进行计算,比如剩余容量、健康状况评估、热平衡控制等。可以利用numpy和pandas库进行数值处理。
```python
import numpy as np
import pandas as pd
def analyze_data(voltage, current, temperature):
soc = calculate_soc(voltage, current)
health_status = check_health(soc, temperature)
return soc, health_status
```
3. **故障诊断与预警**:设置阈值并检测异常情况,例如超过特定范围的电流、电压波动或过热。当条件满足时,触发报警或者采取保护措施。
```python
def fault_detection(soc, health_status):
if soc < MIN_SOC or health_status == 'unhealthy':
raise Alarm('Potential issue detected')
```
4. **电池均衡**:根据电池单元间的不平衡情况进行充电调整,使用算法(如模糊逻辑、PID控制)来控制充电电流。
```python
from fuzzy import ControlSystem
def battery_balancing(currents):
control_system = ControlSystem()
corrected_currents = control_system.run(currents)
return corrected_currents
```
5. **用户界面与日志记录**:通过GUI库(如Tkinter)展示实时数据和警告信息,同时将重要事件写入日志文件。
```python
import tkinter as tk
def display_info(soc, health_status):
root.update()
# 显示在界面上...
with open('bms_log.txt', 'a') as log_file:
log_file.write(f"Time: {datetime.now()}, SOC: {soc}, Health Status: {health_status}\n")
```
阅读全文
相关推荐
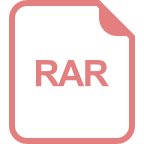
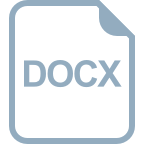
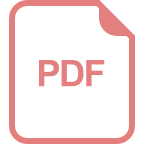
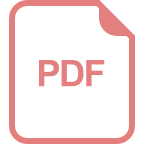
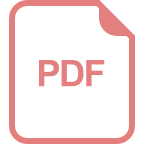
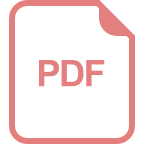
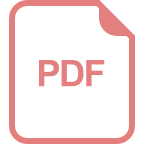
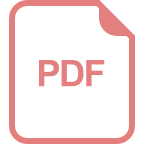
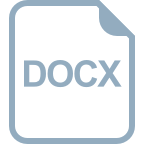
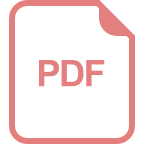
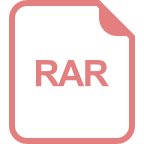
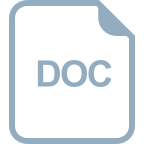
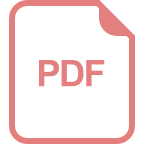
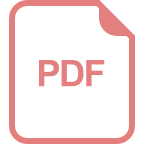
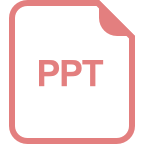
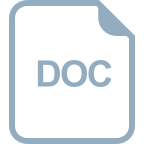
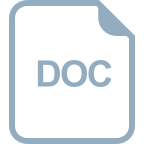
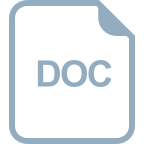
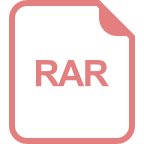