pulp24.3.1库中显示值的代码
时间: 2024-11-06 10:13:43 浏览: 9
Pulp是Python的一个优化库,主要用于线性规划、整数规划和动态规划等问题。如果你提到的`pulp24.3.1`是一个错误版本,因为Pulp的主要版本号通常只有两位(如`2.x`),而不是三位。不过,假设你是指的是Pulp库中的某个特定功能展示变量值,下面是一个基本的例子:
```python
from pulp import LpProblem, LpVariable
# 创建一个优化问题实例
prob = LpProblem("My Problem", LpMaximize)
# 定义一些变量
x = LpVariable(name="x", lowBound=0, upBound=None) # 这里假设x是连续变量
y = LpVariable(name="y", cat='Binary') # y是个二元变量
# 添加约束和目标函数
prob += x + 2*y >= 5 # 示例约束
prob += objective_function(x, y) # 根据实际问题设定目标函数
# 解决问题
status = prob.solve()
# 打印结果
if status == LpStatusOptimal:
print(f"最优解: {x.varValue} (x), {y.varValue} (y)")
else:
print("未找到最优解")
```
在这个例子中,`varValue`属性返回了变量在当前解决方案下的取值。请注意,你需要替换`objective_function`为你实际的问题目标函数。
相关问题
python pulp 库中如何显示Total iterations 的所有迭代过程
在Python的Pulp库中,用于解决线性规划和整数优化等问题时,通常使用LpProblem和相关的求解器函数,比如LpSolverDefault.solve()。默认情况下,Pulp不会显示所有的迭代过程信息,包括总迭代次数。如果你想要跟踪求解过程并查看迭代次数,你需要使用Pulp的一些高级设置或者自定义解决方案。
首先,你需要导入Pulp库和其他必要的模块:
```python
from pulp import LpProblem, LpStatus, PuLP, lpSum
```
然后,你可以创建一个模型,并开启Pulp的详细输出模式,例如使用PuLP.CPLEX作为求解器时,可以设置`CPLEX.setOption('display', 'full')`。但在实际操作中,这可能会输出大量信息,可能会比较混乱,因此通常只在调试阶段这么做:
```python
PuLP.CPLEX.setOption('display', 'full')
```
接着,在求解过程中,Pulp的返回值会包含一些信息,其中包括迭代次数。例如,你可以这样做来获取最终的迭代次数:
```python
problem = LpProblem("My Problem", LpMinimize)
... # 添加变量、约束和目标函数
status = problem.solve()
if status == LpStatusOptimal:
num_iterations = PuLP.LpSolveIterations()
print(f"Total iterations: {num_iterations}")
else:
print("Optimization failed.")
```
在这里,`num_iterations`就是你在解决问题时Pulp所经历的迭代次数。
但是请注意,不是所有求解器都提供详细的迭代信息,而且Pulp默认并不会记录每个迭代的具体信息。如果需要更细致的信息,你可能需要查阅特定求解器的文档。
调用pulp库来重写以上代码
好的,以下是使用pulp库重新编写的代码:
```python
import pulp as lp
import numpy as np
def integer_cutting_plane(c, A, b, bounds):
relaxed_A = A
relaxed_b = b
while True:
lp_model = lp.LpProblem("Integer Cutting Plane", lp.LpMinimize)
# 定义决策变量
x = [lp.LpVariable(f"x{i}", lowBound=bound[0], upBound=bound[1], cat='Integer') for i, bound in enumerate(bounds)]
# 定义目标函数
lp_model += sum(c[i] * x[i] for i in range(len(c)))
# 添加松弛约束
for i in range(len(relaxed_A)):
lp_model += sum(relaxed_A[i][j] * x[j] for j in range(len(x))) <= relaxed_b[i]
lp_model.solve()
if lp_model.status == lp.LpStatusOptimal:
return np.array([int(x[i].varValue) for i in range(len(x))])
new_constraint = [relaxed_A[i] @ [x[j].varValue for j in range(len(x))] <= relaxed_b[i] for i in range(len(relaxed_A))]
relaxed_A = np.vstack((relaxed_A, new_constraint))
relaxed_b = np.append(relaxed_b, np.inf)
def get_bounds():
return [(0, None), (0, None)]
def get_c():
return np.array([40, 90])
def get_A():
return np.array([[-9, -7], [-7, -20]])
def get_b():
return np.array([-56, -70])
if __name__ == '__main__':
bounds = get_bounds()
relaxed_A = get_A()
relaxed_b = get_b()
integer_cutting_plane(get_c(), relaxed_A, relaxed_b, bounds)
```
在这个代码中,我们使用pulp库来定义和解决整数线性规划问题。我们首先创建一个线性规划模型`lp_model`,然后定义决策变量、目标函数和约束条件。在每次迭代中,我们调用`lp_model.solve()`来求解整数线性规划问题,并根据求解结果判断是否找到了整数可行解。
希望这次的代码能够满足你的需求。如果有任何进一步的问题,请随时提问。
阅读全文
相关推荐
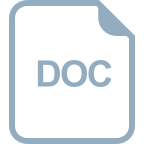
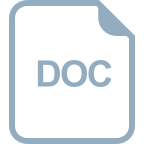
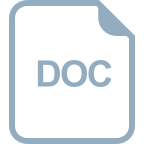
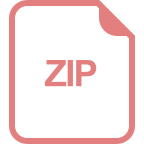











