怎么敲出发出请求 提交页面,Java web,eclipse
时间: 2024-10-13 16:17:12 浏览: 22
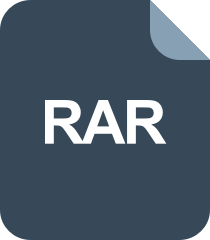
java web 一个简陋的网站页面
在Java Web应用中,如果你想从Eclipse环境中发出HTTP请求并提交页面,通常有几种方法:
1. **使用内置工具:** Eclipse有一个内置的Web浏览器插件(有时称为“Deployment Client”),可以在浏览器窗口中发送GET或POST请求。右键点击部署的WAR文件或项目的src/main/webapp目录,选择“Run As” -> “Deploy on Server”,然后在弹出的界面中,你可以看到一个选项用于发起HTTP请求。
2. **使用浏览器插件:** 另一种选择是安装像Postman这样的Chrome浏览器插件,这允许你在浏览器控制台中方便地构造和发送HTTP请求。在Eclipse中,你可以在命令行工具或终端通过浏览器打开Postman,然后导入服务器地址进行测试。
3. **编写Java代码:** 使用标准库如HttpURLConnection或者第三方库如Apache HttpClient或OkHttp,可以编写Java代码来发起HTTP请求。首先,在Eclipse中创建一个新的Java类,然后添加以下代码片段作为示例:
```java
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class HttpRequestExample {
public static void main(String[] args) throws Exception {
URL url = new URL("http://your-url.com/submit-page");
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
// 设置请求方法(POST)
connection.setRequestMethod("POST");
// 设置请求头,如果有需要的话(如设置Content-Type)
connection.setRequestProperty("Content-Type", "application/x-www-form-urlencoded");
String data = "key=value&another_key=another_value";
byte[] input = data.getBytes("UTF-8");
connection.setDoOutput(true);
connection.getOutputStream().write(input);
int responseCode = connection.getResponseCode();
BufferedReader in = new BufferedReader(new InputStreamReader(connection.getInputStream()));
System.out.println("Response Code : " + responseCode);
String output;
while ((output = in.readLine()) != null) {
System.out.println(output);
}
in.close();
connection.disconnect();
}
}
```
记得替换`http://your-url.com/submit-page`为实际的URL,并按需修改请求头和参数。
**相关问题--:**
1. 如何处理POST请求的表单数据?
2. 使用上述方法发送请求时,如何处理可能出现的异常?
3. 如何在Eclipse中配置HttpURLConnection以处理cookies?
阅读全文
相关推荐
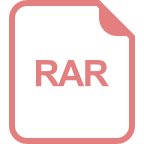
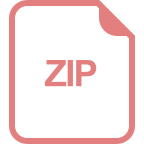
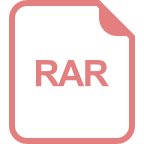
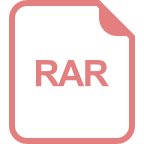
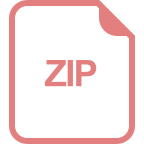
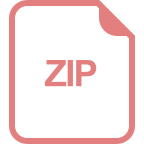
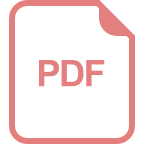
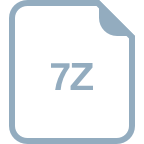
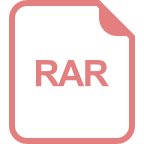
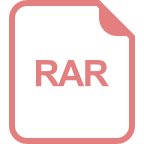
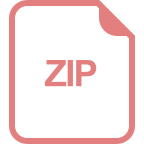
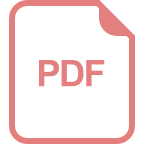
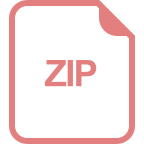
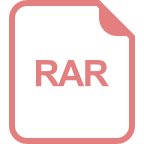
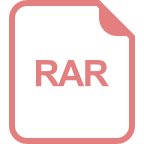
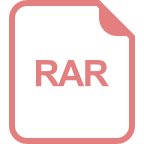
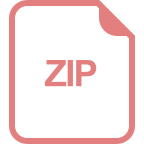
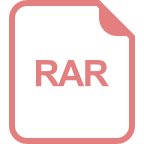