吸烟者问题源码实现c语言操作系统上机
时间: 2023-12-21 18:29:49 浏览: 46
根据提供的引用内容,吸烟者问题是一个经典的同步问题,通常用于操作系统的并发编程中。下面是一个C语言实现的吸烟者问题源码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#include <semaphore.h>
#include <unistd.h>
#define N 3 // 烟草、纸、胶水三种材料
#define LEFT (i-1+3)%3 // 左边的材料
#define RIGHT (i+1)%3 // 右边的材料
sem_t tobacco, paper, glue, agent; // 信号量
void *smoker(void *arg) {
int i = *(int *)arg;
while (1) {
sem_wait(&agent); // 等待中介者放置材料
if (i == 0) {
sem_wait(&paper);
sem_wait(&glue);
printf("Smoker %d is smoking...\n", i);
sleep(1);
sem_post(&agent);
sem_post(&tobacco);
} else if (i == 1) {
sem_wait(&tobacco);
sem_wait(&glue);
printf("Smoker %d is smoking...\n", i);
sleep(1);
sem_post(&agent);
sem_post(&paper);
} else {
sem_wait(&tobacco);
sem_wait(&paper);
printf("Smoker %d is smoking...\n", i);
sleep(1);
sem_post(&agent);
sem_post(&glue);
}
}
}
void *agent_func(void *arg) {
while (1) {
sleep(1);
int r = rand() % 3;
if (r == 0) {
sem_post(&tobacco);
sem_post(&paper);
} else if (r == 1) {
sem_post(&tobacco);
sem_post(&glue);
} else {
sem_post(&paper);
sem_post(&glue);
}
sem_wait(&agent);
}
}
int main() {
pthread_t tid[4];
int index[3] = {0, 1, 2};
sem_init(&tobacco, 0, 0);
sem_init(&paper, 0, 0);
sem_init(&glue, 0, 0);
sem_init(&agent, 0, 1);
pthread_create(&tid[0], NULL, smoker, &index[0]);
pthread_create(&tid[1], NULL, smoker, &index[1]);
pthread_create(&tid[2], NULL, smoker, &index[2]);
pthread_create(&tid[3], NULL, agent_func, NULL);
pthread_join(tid[0], NULL);
pthread_join(tid[1], NULL);
pthread_join(tid[2], NULL);
pthread_join(tid[3], NULL);
sem_destroy(&tobacco);
sem_destroy(&paper);
sem_destroy(&glue);
sem_destroy(&agent);
return 0;
}
```
上述代码中,使用了4个线程,其中3个线程代表3个吸烟者,另一个线程代表中介者。每个吸烟者需要等待中介者放置两种材料,然后才能开始吸烟。中介者会随机放置两种材料,然后通知吸烟者开始吸烟。在代码中,使用了信号量来实现线程之间的同步。
相关推荐
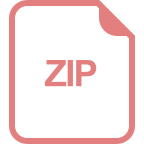














