relaycommand如何写异步
时间: 2024-12-12 12:32:59 浏览: 19
`RelayCommand`通常是指Unity中的一个命令模式(Command Pattern)实现,用于处理游戏中的事件或操作。在编写异步版本的`RelayCommand`时,你需要考虑将任务放在一个可以取消的等待操作中,例如`Task`或者`IAsyncDisposable`。下面是一个简单的示例:
```csharp
using UnityEngine;
using UnityEngine.Events;
public class AsyncRelayCommand : IAsyncDisposable {
private UnityAction _executeMethod;
private bool _isExecuting = false;
private TaskCompletionSource<bool> _taskCompletionSource;
public AsyncRelayCommand(UnityAction executeMethod) {
_executeMethod = executeMethod;
}
public async ValueTask DisposeAsync() {
// 如果命令正在执行,取消它
if (_isExecuting && _taskCompletionSource != null) {
_taskCompletionSource.TrySetCanceled();
}
}
public void Execute() {
if (!_isExecuting) {
_isExecuting = true;
_taskCompletionSource = new TaskCompletionSource<bool>();
StartCoroutine(RunExecuteMethod());
} else {
Debug.LogError("Command is already executing.");
}
}
IEnumerator RunExecuteMethod() {
try {
_executeMethod.Invoke();
_taskCompletionSource.SetResult(true); // 命令执行成功
} catch (Exception ex) {
Debug.LogError(ex.Message);
_taskCompletionSource.SetException(ex); // 命令执行失败
} finally {
_isExecuting = false; // 无论结果如何,标记为已执行完毕
}
}
}
// 使用示例:
private AsyncRelayCommand myCommand;
...
myCommand = new AsyncRelayCommand(() => SomeLongRunningTask());
myCommand.Execute().Wait(); // 异步等待命令完成
```
在这个例子中,当`Execute`方法被调用时,会创建一个新的异步任务,并在其完成后调用`_executeMethod`。如果命令已经在执行中,则忽略新的请求并记录警告。
阅读全文
相关推荐










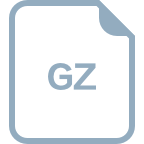

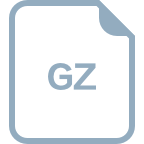
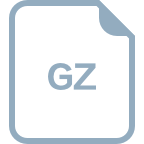
