qt 如何防止快速点击按钮导致信号重复触发
时间: 2024-09-28 20:15:33 浏览: 126
在Qt中,防止快速点击按钮导致信号多次触发(也称为“debouncing”或“防抖处理”),通常是为了避免程序对短时间内连续发生的事件做出不必要的响应。你可以通过以下几种方式来实现:
1. **使用`QTimer`**:当用户点击按钮后,启动一个定时器,设置一个合理的延时时间(比如200毫秒到500毫秒)。如果在这段时间内再次点击,计时器会覆盖上一次的信号发射,仅在定时器到期后发送一次信号。
```cpp
connect(button, &QPushButton::clicked, this, [this](bool checked) {
QTimer::singleShot(300, [this]() { yourSignalHandler(); });
});
```
2. **利用`SLOT()`和`SLOT delayingSlot()`**:Qt提供了一种特殊的槽函数`delayingSlot()`,它可以延迟执行信号处理。例如:
```cpp
connect(button, &QPushButton::clicked, this, SLOT(delayingClick()));
void delayingClick() {
QTimer::singleShot(300, this, SLOT(yourSignalHandler()));
}
```
3. **信号缓冲机制**:Qt的一些信号默认支持短时间内的信号抑制,如`QSignalMapper`。可以创建一个信号映射器并使用`block()`和`unblock()`方法来暂时阻止信号直到下一次点击间隔过去。
```cpp
QSignalMapper mapper;
mapper.setMapping(&button, 0);
connect(&button, &QPushButton::clicked, &mapper, &QSignalMapper::map);
connect(&mapper, &QSignalMapper::mapped, this, &YourClass::yourSignalHandler);
// 模板信号,每次接收到点击,暂停一段时间
void yourSignalHandler() {
if (mapper.map() == 0) {
QTimer::singleShot(300, this, &YourClass::yourSignalHandler);
}
}
```
阅读全文
相关推荐
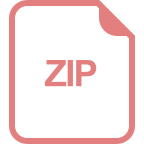
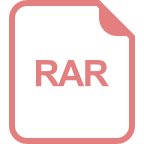
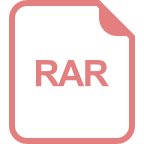
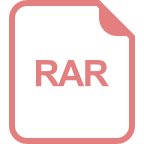
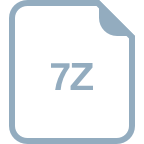
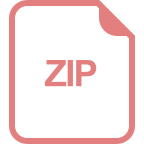
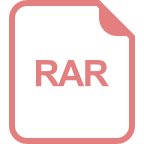
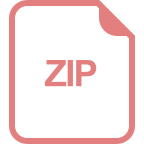
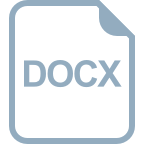
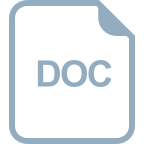
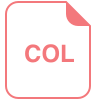
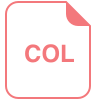
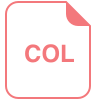
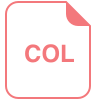
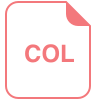
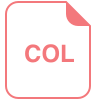
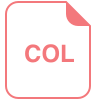
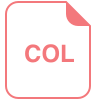
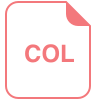