java爱心代码跳动可运行
时间: 2024-07-01 13:00:44 浏览: 7
在Java中,实现一个爱心代码跳动效果通常涉及到动画或者GUI编程。这里我们可以用Swing库来创建一个简单的爱心形状,并通过定时器让它看起来像是在跳动。以下是一个基本的示例代码:
```java
import javax.swing.*;
import java.awt.geom.Path2D;
import java.awt.geom.Point2D;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class HeartBouncer extends JFrame {
private JLabel heartLabel;
private Timer timer;
public HeartBounce() {
setSize(400, 400);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLayout(new FlowLayout());
// 创建爱心形状
Shape heartShape = createHeartShape();
// 创建label并设置背景为透明
heartLabel = new JLabel(new ShapeIcon(heartShape), SwingConstants.CENTER);
heartLabel.setBackground(new Color(0, 0, 0, 0)); // 透明背景
// 添加到窗口
add(heartLabel);
// 初始化定时器
timer = new Timer(50, new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
Point2D.Float position = heartLabel.getIcon().getIconRect().getLocation();
position.y += 2; // 每次跳跃增加2个像素高度
if (position.y > getHeight()) {
position.y = 0; // 当超出屏幕顶部时重置
}
heartLabel.setBounds(position.x, position.y, heartLabel.getWidth(), heartLabel.getHeight());
}
});
timer.start();
}
private Shape createHeartShape() {
Path2D.Float path = new Path2D.Float();
path.moveTo(-50, -30);
path.lineTo(-40, -30);
path.lineTo(-40, -40);
path.lineTo(0, -40);
path.lineTo(0, -50);
path.lineTo(10, -50);
path.lineTo(10, -60);
path.lineTo(20, -60);
path.lineTo(20, -70);
path.lineTo(30, -70);
path.lineTo(30, -80);
path.lineTo(40, -80);
path.lineTo(40, -90);
path.lineTo(50, -90);
path.lineTo(50, -80);
path.lineTo(60, -80);
path.lineTo(60, -70);
path.lineTo(70, -70);
path.lineTo(70, -60);
path.lineTo(80, -60);
path.lineTo(80, -50);
path.lineTo(90, -50);
path.lineTo(90, -40);
path.lineTo(100, -40);
path.lineTo(100, -30);
path.closePath();
return path;
}
public static void main(String[] args) {
SwingUtilities.invokeLater(new Runnable() {
@Override
public void run() {
new HeartBounce().setVisible(true);
}
});
}
}
class ShapeIcon implements Icon {
private Shape shape;
public ShapeIcon(Shape shape) {
this.shape = shape;
}
@Override
public void paintIcon(Component c, Graphics g, int x, int y) {
Graphics2D g2d = (Graphics2D) g.create();
g2d.setPaint(Color.RED);
g2d.fill(shape);
g2d.dispose();
}
@Override
public int getIconWidth() {
return (int) Math.ceil(shape.getBounds().getWidth());
}
@Override
public int getIconHeight() {
return (int) Math.ceil(shape.getBounds().getHeight());
}
}
```
相关推荐
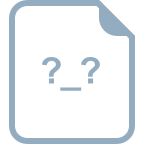
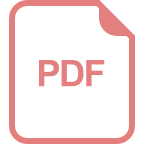
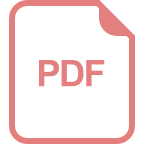













