用c语言写一个基于线性表的图书信息管理代码
时间: 2024-09-27 08:14:50 浏览: 77
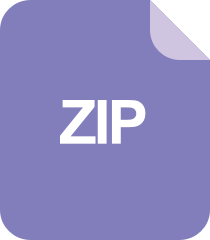
基于线性表的图书管理系统 源代码 顺序表&链表
在C语言中编写一个简单的图书信息管理程序,我们可以创建一个结构体表示图书信息,如书名、作者、出版社等,并利用数组或链表作为数据结构存储。这里我们使用链表为例,因为它可以动态添加或删除图书信息。
首先,定义一个包含图书信息的结构体:
```c
typedef struct Book {
char title[50];
char author[50];
char publisher[50];
int id;
} Book;
```
然后,创建一个链表结构以及相关的操作函数:
```c
#include <stdio.h>
#include <stdlib.h>
// 链表节点结构体
typedef struct Node {
Book book;
struct Node* next;
} Node;
// 创建新节点
Node* create_node(Book book) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (!newNode) {
printf("Memory allocation failed.\n");
return NULL;
}
newNode->book = book;
newNode->next = NULL;
return newNode;
}
// 添加图书到链表
void add_book(Node** head, Book book) {
Node* newNode = create_node(book);
if (*head == NULL) {
*head = newNode;
} else {
Node* current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = newNode;
}
}
// 打印链表中的所有图书
void print_books(Node* head) {
Node* current = head;
while (current != NULL) {
printf("ID: %d\nTitle: %s\nAuthor: %s\nPublisher: %s\n",
current->book.id, current->book.title, current->book.author, current->book.publisher);
current = current->next;
}
}
int main() {
// 初始化链表
Node* head = NULL;
// 添加书籍示例
Book book1 = {"图书1", "作者1", "出版社1", 1};
add_book(&head, book1);
// 打印所有书籍
print_books(head);
return 0;
}
```
阅读全文
相关推荐



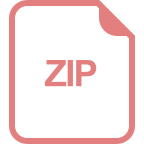
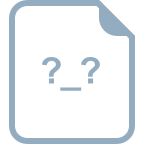
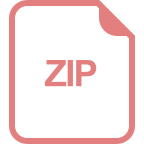

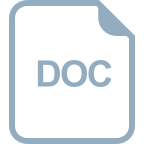
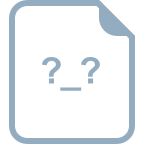
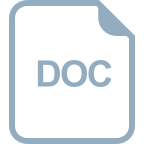
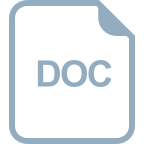
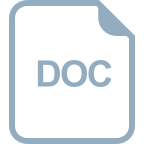



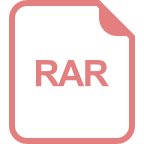
