java easypoi 设置某列左对齐
时间: 2023-09-02 15:04:26 浏览: 153
要设置某列左对齐,可以使用Java的easypoi库来实现。easypoi是一个用于操作excel的开源库,可以方便地进行excel文件的读取和写入。
首先,我们需要导入easypoi库的相关依赖。在项目的pom.xml文件中,添加以下依赖:
```xml
<dependency>
<groupId>cn.afterturn</groupId>
<artifactId>easypoi-base</artifactId>
<version>3.3.0</version>
</dependency>
<dependency>
<groupId>cn.afterturn</groupId>
<artifactId>easypoi-annotation</artifactId>
<version>3.3.0</version>
</dependency>
```
接下来,在代码中通过easypoi来读取excel文件,并设置某列左对齐。假设我们要设置第二列左对齐,代码如下所示:
```java
import cn.afterturn.easypoi.excel.ExcelImportUtil;
import cn.afterturn.easypoi.excel.entity.ImportParams;
import cn.afterturn.easypoi.excel.entity.params.ExcelExportEntity;
import cn.afterturn.easypoi.excel.export.ExcelExportUtil;
import cn.afterturn.easypoi.excel.export.styler.IExcelExportStyler;
import cn.afterturn.easypoi.excel.export.styler.StylerUtils;
import cn.afterturn.easypoi.excel.export.styler.theme.DefaultTheme;
import cn.afterturn.easypoi.handler.inter.IExcelDataHandler;
import cn.afterturn.easypoi.handler.inter.IExcelDictHandler;
import cn.afterturn.easypoi.handler.inter.IExcelExportServer;
import org.apache.poi.ss.usermodel.*;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.util.ArrayList;
import java.util.List;
public class ExcelExample {
public static void main(String[] args) {
try {
// 读取excel文件
InputStream is = new FileInputStream("path_to_excel_file");
ImportParams params = new ImportParams();
List<Object> dataList = ExcelImportUtil.importExcel(is, Object.class, params);
// 设置左对齐样式
IExcelExportStyler styler = new StylerUtils();
CellStyle cellStyle = styler.getStyles(null).get(StylerUtils.LEFT_ALIGNMENT_STYLE);
List<ExcelExportEntity> exportEntityList = new ArrayList<>();
for (int i = 0; i < dataList.get(0).getClass().getDeclaredFields().length; i++) {
ExcelExportEntity exportEntity = new ExcelExportEntity("Column " + (i+1), "field" + (i+1));
exportEntity.setStyle(cellStyle);
exportEntityList.add(exportEntity);
}
// 导出excel文件
Workbook workbook = ExcelExportUtil.exportBigExcel(new ExportParams(), exportEntityList, dataList);
FileOutputStream fos = new FileOutputStream("path_to_output_excel_file");
workbook.write(fos);
workbook.close();
fos.close();
is.close();
System.out.println("Excel exported successfully!");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
以上代码中,我们首先通过easypoi的`ExcelImportUtil`来读取excel文件,并将数据保存在`dataList`中。接着,我们定义了一个`IExcelExportStyler`对象用于获取样式,然后通过设置`CellStyle`对象的样式来实现左对齐。最后,使用`ExcelExportUtil`将数据和样式一起导出到excel文件中。
请注意,代码中的`path_to_excel_file`和`path_to_output_excel_file`需要替换为您实际的文件路径。
通过以上步骤,就可以使用Java的easypoi库来设置某列左对齐了。
相关推荐
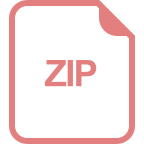
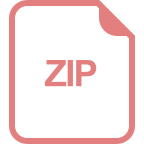














