index(可选):当前元素在数组中的索引(从0开始或从initialValue指定的位置开始)。array(可选):调用 reduce 方法的原数组。
时间: 2024-09-09 09:04:06 浏览: 46
`index` 参数在 JavaScript 的 `Array.prototype.reduce()` 方法中是可选的,它表示当前正在处理的数组元素的索引。这个索引是从 0 开始计算的,如果你没有提供初始值 (`initialValue`),那么它将从数组的第一个元素开始递增。如果提供了 `initialValue`,则索引会从那个位置开始。
`array` 参数则是必需的,它是你要对之应用累加器函数 (reducer) 的数组。`reduce()` 方法会对数组中的每个元素依次应用提供的函数,最终返回一个单一的累积结果。
例如:
```javascript
let numbers = [1, 2, 3, 4, 5];
let sum = numbers.reduce((accumulator, currentValue, index) => accumulator + currentValue, 0);
console.log(sum); // 输出:15
```
在这个例子中,`index` 参数用于跟踪当前处理的数字在数组中的位置,而 `array` 参数是整个数字数组 `[1, 2, 3, 4, 5]`。
相关问题
在js中数组的reduce方法
在JavaScript中,`Array.prototype.reduce()`是一个高阶函数,用于对数组的所有元素执行一个由您提供的累加器函数(accumulator function),将每个元素减少到单个值。这个方法通常用于求和、查找最大值、最小值,或者基于特定规则计算数组的摘要信息。
它的基本语法如下:
```javascript
array.reduce(callback[, initialValue])
```
- `callback`: 函数,接受四个参数:当前元素(currentValue)、累计结果(previousValue,初始值为`initialValue`或数组的第一个元素,如果提供的话)、当前索引(index)以及原数组(array)。
- `initialValue` (可选): 初始累加器值,默认为数组的第一个元素。如果没有指定,则从数组的第一个元素开始。
回调函数的一般形式如下:
```javascript
function accumulator(currentValue, accumulatedValue, currentIndex, array) {
// 对当前元素和累计值进行操作并返回新的累积值
}
```
举个例子,如果我们有一个数组,我们可以用它来计算所有数的总和:
```javascript
let numbers = [1, 2, 3, 4, 5];
let sum = numbers.reduce(function(accumulator, currentValue) {
return accumulator + currentValue;
}, 0); // 初始化值为0
console.log(sum); // 输出:15
```
数组api-findIndex filter reduce
1. findIndex() - 返回数组中满足条件的第一个元素的索引,如果没有符合条件的元素,则返回 -1。
语法:array.findIndex(callback(element[, index[, array]])[, thisArg])
参数:
callback:用来测试每个元素的函数,返回 true 表示当前元素符合条件,false 则不符合。
element:当前遍历到的元素。
index:当前遍历到的元素的索引。
array:数组本身。
thisArg:可选,执行 callback 函数时使用的 this 值。
示例:
const arr = [1, 2, 3, 4, 5];
const index = arr.findIndex((element) => element > 3);
console.log(index); // 3
2. filter() - 返回一个新数组,其中包含原数组中所有符合条件的元素。
语法:array.filter(callback(element[, index[, array]])[, thisArg])
参数:
callback:用来测试每个元素的函数,返回 true 表示当前元素符合条件,false 则不符合。
element:当前遍历到的元素。
index:当前遍历到的元素的索引。
array:数组本身。
thisArg:可选,执行 callback 函数时使用的 this 值。
示例:
const arr = [1, 2, 3, 4, 5];
const newArr = arr.filter((element) => element > 3);
console.log(newArr); // [4, 5]
3. reduce() - 通过对数组中的每个元素进行归纳操作,将数组归纳为单个值。
语法:array.reduce(callback(previousValue, currentValue[, index[, array]])[, initialValue])
参数:
callback:归纳操作的函数,包含两个参数:
previousValue:先前归纳操作的结果,或者在第一次调用时是 initialValue。
currentValue:当前遍历到的元素。
index:当前遍历到的元素的索引。
array:数组本身。
initialValue:可选,作为归纳操作的初始值。
示例:
const arr = [1, 2, 3, 4, 5];
const sum = arr.reduce((previousValue, currentValue) => previousValue + currentValue, 0);
console.log(sum); // 15
阅读全文
相关推荐
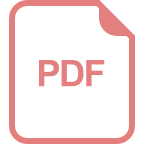
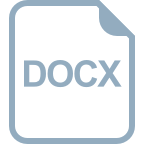
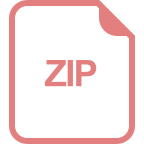
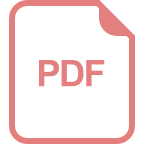
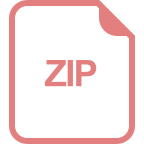
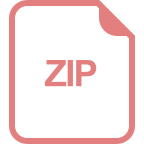
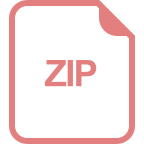
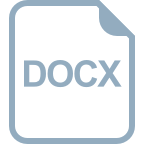
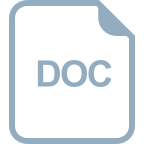
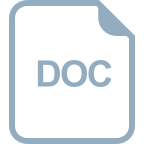
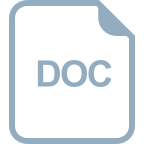



