c++中message_check 函数定义方法
时间: 2024-09-25 14:03:30 浏览: 32
在 C++ 中,`message_check` 这个函数名并不是标准库的一部分,也不是所有项目都会有的自定义函数。通常情况下,它可能是某个特定框架、库或者是某个项目中的一个私有成员函数,用于检查消息的合法性或者某些条件。
例如,在一些通信协议处理或者错误处理场景中,可能会有一个名为 `message_check` 的函数,它的作用可能是验证接收到的消息是否符合预期格式,或者检查消息内容是否存在错误。具体的实现会依赖于项目的具体设计和需求:
```cpp
// 假设这是一个简单的示例
bool message_check(const Message& msg) {
if (msg.header.checksum != compute_checksum(msg.content)) {
std::cerr << "Message checksum mismatch!" << std::endl;
return false;
}
// 可能还会检查其他字段或条件...
return true;
}
// 计算消息校验和的方法
uint32_t compute_checksum(const std::vector<uint8_t>& data) {
// 实现具体的校验算法...
}
```
如果你想要了解更具体的情况,需要提供更多的上下文信息或者是在哪个库或框架中遇到这个函数。如果这只是一个练习题或者假设情况,那么你需要编写这样的函数,并根据实际业务逻辑去实现相应的功能。
相关问题
if (is_blacklisted(pl_name, blacklist, whitelist)) { ROS_INFO_STREAM("Plugin " << pl_name << " blacklisted"); return; } try { auto plugin = plugin_loader.createInstance(pl_name); ROS_INFO_STREAM("Plugin " << pl_name << " loaded"); for (auto &info : plugin->get_subscriptions()) { auto msgid = std::get<0>(info); auto msgname = std::get<1>(info); auto type_hash_ = std::get<2>(info); std::string log_msgname; if (is_mavlink_message_t(type_hash_)) log_msgname = utils::format("MSG-ID (%u) <%zu>", msgid, type_hash_); else log_msgname = utils::format("%s (%u) <%zu>", msgname, msgid, type_hash_); ROS_DEBUG_STREAM("Route " << log_msgname << " to " << pl_name); auto it = plugin_subscriptions.find(msgid); if (it == plugin_subscriptions.end()) { // new entry ROS_DEBUG_STREAM(log_msgname << " - new element"); plugin_subscriptions[msgid] = PluginBase::Subscriptions{{info}}; } else { // existing: check handler message type bool append_allowed = is_mavlink_message_t(type_hash_); if (!append_allowed) { append_allowed = true; for (auto &e : it->second) { auto t2 = std::get<2>(e); if (!is_mavlink_message_t(t2) && t2 != type_hash_) { ROS_ERROR_STREAM(log_msgname << " routed to different message type (hash: " << t2 << ")"); append_allowed = false; } } } if (append_allowed) { ROS_DEBUG_STREAM(log_msgname << " - emplace"); it->second.emplace_back(info); } else ROS_ERROR_STREAM(log_msgname << " handler dropped because this ID are used for another message type"); } } plugin->initialize(mav_uas); loaded_plugins.push_back(plugin); ROS_INFO_STREAM("Plugin " << pl_name << " initialized"); } catch (pluginlib::PluginlibException &ex) { ROS_ERROR_STREAM("Plugin " << pl_name << " load exception: " << ex.what()); } }
这是一段C++代码,用于加载和初始化插件,并将其与消息路由器相关联。首先,它使用“is_blacklisted”函数检查插件是否在黑名单或白名单中。如果插件在黑名单中,则不会加载。然后,它使用“plugin_loader”对象创建插件实例,并调用“get_subscriptions”函数获取插件支持的订阅消息类型。对于每个订阅类型,它将其与插件名称相关联,并将其添加到“plugin_subscriptions”映射中。如果消息ID已经存在于“plugin_subscriptions”中,则会检查处理程序的消息类型是否与现有类型匹配。如果匹配,则将处理程序添加到现有消息ID的列表中。如果不匹配,则会记录错误消息并跳过处理程序。最后,它调用“initialize”函数初始化插件,并将其添加到“loaded_plugins”列表中。
在ros中用c++实现给定的topic是否有数据,代码中不需要提前定义回调函数
在 ROS 中,可以使用 roscpp 库来实现给定的 topic 是否有数据。以下是一个示例代码,不需要提前定义回调函数,可以检测指定的 topic 是否有数据:
```c++
#include <ros/ros.h>
#include <std_msgs/String.h>
int main(int argc, char** argv)
{
ros::init(argc, argv, "topic_check");
ros::NodeHandle nh;
ros::Subscriber sub = nh.subscribe<std_msgs::String>("my_topic", 10, [](const std_msgs::String::ConstPtr& msg) {
ROS_INFO("Received message: %s", msg->data.c_str());
});
while (ros::ok()) {
ros::spinOnce();
if (sub.getNumPublishers() > 0) {
ROS_INFO("Topic has data");
} else {
ROS_INFO("Topic has no data");
}
ros::Duration(1.0).sleep();
}
return 0;
}
```
在上面的代码中,我们定义了一个订阅器 `sub`,它订阅了名为 `my_topic` 的 topic,并且指定了一个 lambda 表达式作为回调函数,当有新的消息到达时,会打印消息内容。然后,在一个 while 循环中,我们不断调用 `ros::spinOnce()` 函数来处理消息,然后使用 `sub.getNumPublishers()` 函数来检查该 topic 是否有数据。如果 `getNumPublishers()` 返回值大于 0,则表示该 topic 有数据,否则表示该 topic 没有数据。最后,我们使用 `ros::Duration(1.0).sleep()` 函数来让程序休眠 1 秒钟,然后继续执行下一次循环。
阅读全文
相关推荐
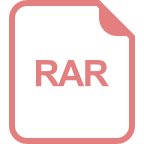
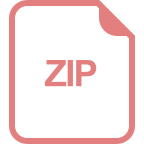
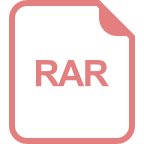
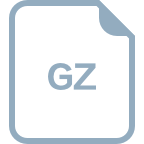
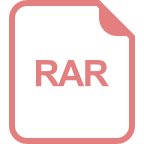
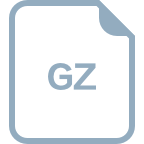
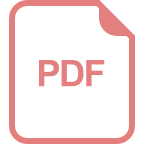
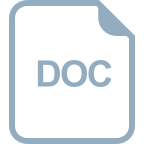
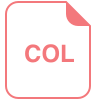
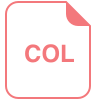
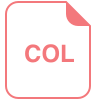
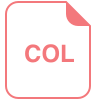
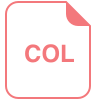
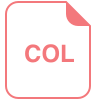
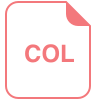
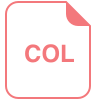
