python作业要求:获得天气数据,生成简报,格式如下
时间: 2024-10-08 21:07:13 浏览: 53
在Python作业中,如果需要获取天气数据并生成简报,通常会涉及以下几个步骤:
1. **数据获取**:首先,你需要使用一个天气API(如OpenWeatherMap、AccuWeather等)来获取实时或预报的天气信息。这通常需要安装相应的库,比如`requests`用于发送HTTP请求,`json`处理API返回的数据。
```python
import requests
api_key = "your_api_key"
url = f"http://api.openweathermap.org/data/2.5/weather?q={city}&appid={api_key}"
response = requests.get(url)
data = response.json()
```
2. **数据解析**:对获取到的JSON数据进行解析,提取出温度、湿度、风速、天气状况等关键信息。
```python
temperature = data["main"]["temp"]
humidity = data["main"]["humidity"]
wind_speed = data["wind"]["speed"]
weather_description = data["weather"][0]["description"]
```
3. **生成简报**:将数据格式化成易于理解的文本,例如:
```python
report = f"城市:{data['name']}\n当前气温:{temperature}°C\n湿度:{humidity}%\n风速:{wind_speed} m/s\n天气描述:{weather_description}"
```
4. **输出结果**:最后将简报打印到控制台或保存到文件中。
```python
print(report)
# 或者
with open('weather_report.txt', 'w') as file:
file.write(report)
```
阅读全文
相关推荐















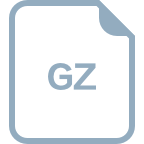
