以此用c++编写代码
时间: 2024-09-19 10:17:01 浏览: 29
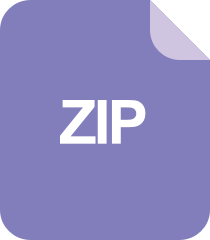
C++ 垃圾代码生成器

在C++中编写电动车租赁管理系统的一个简化示例,我们首先创建一些基本类,并提供一些核心功能。假设我们只关注租赁操作,这里是一个简单的例子:
```cpp
#include <iostream>
#include <string>
class User {
public:
int id;
std::string name;
// 用户注册
void registerUser(int id, const std::string& name) {
this->id = id;
this->name = name;
std::cout << "User " << id << " registered as " << name << std::endl;
}
// 登录验证
bool login(const std::string& password) {
// 简单的密码验证,实际应用中应更复杂
return name == "test" && password == "password";
}
};
class Vehicle {
private:
std::string vehicleId;
bool isAvailable;
public:
// 添加车辆信息
void addVehicle(const std::string& vehicleId) {
this->vehicleId = vehicleId;
isAvailable = true;
std::cout << "Vehicle added with ID: " << vehicleId << std::endl;
}
// 检查车辆是否可用
bool isVehicleAvailable() const {
return isAvailable;
}
// 租赁车辆
void rentVehicle() {
if (isAvailable) {
isAvailable = false;
std::cout << "Vehicle " << vehicleId << " rented by a user." << std::endl;
} else {
std::cout << "Vehicle not available for rental." << std::endl;
}
}
};
class RentalSystem {
private:
User* user;
Vehicle* vehicle;
public:
RentalSystem(User* u, Vehicle* v) : user(u), vehicle(v) {}
void rentalProcess() {
user->login("password"); // 用户登录
if (user->isLoggedIn()) { // 判断用户是否已登录
vehicle->rentVehicle(); // 租车操作
}
}
};
int main() {
User userObj;
Vehicle vehicleObj;
RentalSystem system(&userObj, &vehicleObj);
// 注册用户
userObj.registerUser(1, "test");
// 初始化系统
system.rentalProcess();
return 0;
}
```
这个例子展示了如何创建用户和车辆类,以及在租赁系统中进行基本的租赁操作。请注意,这只是一个简化的模型,实际项目中需要考虑更多的细节,如错误处理、数据持久化和并发控制等。
阅读全文
相关推荐
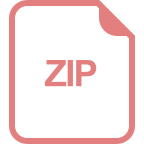
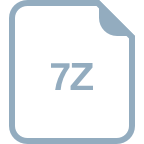
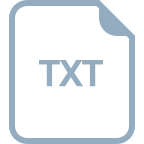

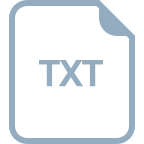
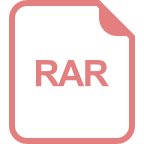
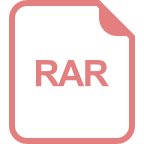
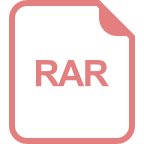
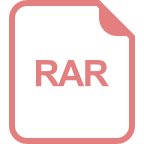
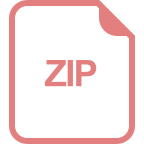
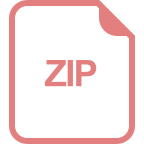
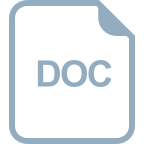
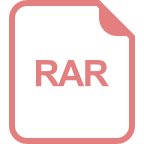
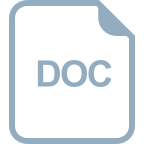



