2. (简答题) 银行账户分为活期和定期两种存款方式,不同存款方式的利息不同。计算利息分为2个步骤:(1)确定账户类型;(2)确定利息。画出银行账户UML图,然后编写程序实现银行账户管理,一种测试结果如下图所示。提示:采用模板模式设计。
时间: 2024-12-12 21:22:11 浏览: 20
在Java中,我们可以使用UML(统一建模语言)来表示银行账户的不同类型以及它们之间的关系。对于这个问题,我们首先需要创建一个抽象的`BankAccount`接口,包含通用的方法如存款、取款和计算利息。然后为活期和定期这两种类型的账户定义具体类,继承自`BankAccount`接口并覆盖计算利息的方法。
这里是一个简单的UML类图描述:
```plaintext
+-------------------+
| BankAccount |
+-------------------+
| -balance |
| +deposit(amount) |
| +withdraw(amount) |
| +calculateInterest()|
+-------------------+
+---------------------+
| SavingsAccount |
+---------------------+
| -interestRate |
| +specificCalculate()|
+---------------------+
+--------------------+
| CheckingAccount|
+--------------------+
| -interestRate |
| +specificCalculate()|
+--------------------+
```
其中,`SavingsAccount`和`CheckingAccount`是具体的银行账户类型,它们都继承自`BankAccount`并重写了`calculateInterest()`方法以提供特定的利息计算逻辑。
接下来,我们按照模板模式设计实现这个类结构:
```java
// 模板类或接口
public abstract class BankAccount {
protected double balance;
public void deposit(double amount) {
// 存款操作
balance += amount;
}
public void withdraw(double amount) {
// 取款操作
if (amount <= balance) {
balance -= amount;
} else {
throw new InsufficientFundsException();
}
}
// 抽象方法,每个子类需要实现
public abstract double calculateInterest();
}
// 具体类1 - 活期账户
public class CheckingAccount extends BankAccount {
private static final double INTEREST_RATE_CHECKING = 0.01; // 假设活期利率
@Override
public double calculateInterest() {
return INTEREST_RATE_CHECKING * balance;
}
}
// 具体类2 - 定期账户
public class SavingsAccount extends BankAccount {
private static final double INTEREST_RATE_SAVINGS = 0.05; // 假设定期利率
@Override
public double calculateInterest() {
return INTEREST_RATE_SAVINGS * balance;
}
}
```
至于测试代码,你可以创建一个`BankAccountManager`类来管理这些账户,并模拟存款、取款和计算利息的操作:
```java
public class BankAccountManager {
private List<BankAccount> accounts;
public void addAccount(BankAccount account) {
accounts.add(account);
}
public void processTransactions(String type, double amount) {
BankAccount account = accounts.stream()
.filter(a -> a.getClass().getSimpleName().equals(type))
.findFirst()
.orElseThrow(() -> new IllegalArgumentException("Invalid account type"));
account.deposit(amount);
System.out.println("After deposit: " + account.balance());
double interest = account.calculateInterest();
System.out.println("Interest earned: " + interest);
}
}
```
测试结果可以像这样显示:
```plaintext
After deposit: ...
Interest earned: ...
After deposit: ...
Interest earned: ...
```
阅读全文
相关推荐





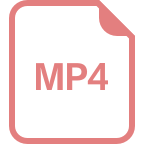